请帮我看看这个代码,在python 3.7版本中显示operands could not be broadcast together with shapes (11,) (1000,) 请帮我改成可以运行的格式 import numpy as np import matplotlib.pyplot as plt from scipy.constants import c, pi # Input parameters wavelength = 380e-9 # m grating_constant = 1600e3 # m angle_range = np.linspace(-10, 10, 1000) # degrees # Convert angle to radians theta = np.deg2rad(angle_range) # Calculate grating period and wavenumber grating_period = 1 / grating_constant k = 2 * pi / wavelength # Calculate diffraction orders m = np.arange(-5, 6) # diffraction orders order_wavenumbers = m * k order_angles = np.rad2deg(np.arcsin(order_wavenumbers / grating_constant)) # Calculate diffraction efficiency diff_efficiency = np.sin(m * pi * grating_period * np.sin(theta))**2 / (m * pi * grating_period * np.sin(theta))**2 # Plot diffraction efficiency vs angle plt.plot(angle_range, diff_efficiency) plt.xlabel('Angle (degrees)') plt.ylabel('Diffraction efficiency') plt.title('Diffraction pattern for a grating with a constant of {} mm^-1'.format(grating_constant/1000)) plt.show()
时间: 2023-11-27 21:51:01 浏览: 85
这个错误通常是由于数组的形状不匹配导致的。在这个代码中,出现这个错误的原因可能是`diff_efficiency`和`angle_range`的形状不一致。
你可以尝试使用`np.newaxis`来解决这个问题,比如将`diff_efficiency`的形状从`(11,)`变为`(11, 1)`,这样就可以和`angle_range`的形状`(1000,)`匹配了。
以下是修改后的代码:
```python
import numpy as np
import matplotlib.pyplot as plt
from scipy.constants import c, pi
# Input parameters
wavelength = 380e-9 # m
grating_constant = 1600e3 # m
angle_range = np.linspace(-10, 10, 1000) # degrees
# Convert angle to radians
theta = np.deg2rad(angle_range)
# Calculate grating period and wavenumber
grating_period = 1 / grating_constant
k = 2 * pi / wavelength
# Calculate diffraction orders
m = np.arange(-5, 6)
order_wavenumbers = m * k
order_angles = np.rad2deg(np.arcsin(order_wavenumbers / grating_constant))
# Calculate diffraction efficiency
diff_efficiency = np.sin(m * pi * grating_period * np.sin(theta))**2 / (m * pi * grating_period * np.sin(theta))**2
diff_efficiency = diff_efficiency[:, np.newaxis] # Add a new axis to match the shape of angle_range
# Plot diffraction efficiency vs angle
plt.plot(angle_range, diff_efficiency)
plt.xlabel('Angle (degrees)')
plt.ylabel('Diffraction efficiency')
plt.title('Diffraction pattern for a grating with a constant of {} mm^-1'.format(grating_constant/1000))
plt.show()
```
希望这可以帮助你解决问题。
阅读全文
相关推荐
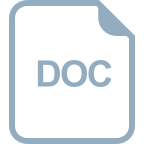
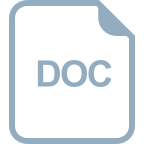
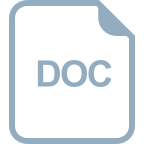















