t1 = time.time() # Note that compactness is defined differently because a grid is not used. Lower compactness for maskSLIC is equivalent segments = seg.slic(img, compactness=10, seed_type='nplace', mask=roi, n_segments=100, recompute_seeds=True, plot_examples=True, enforce_connectivity=True) print("Time: {:.2f} s".format(time.time() - t1)) for segment_id in np.unique(segments): mask = segments == segment_id masked_image = img.copy() masked_image[~mask] = 0 cv2.imwrite(r'I:\18Breakageratecalculation\mask-slic use\maskSLIC-master\result\result6\segment_{}.png'.format(segment_id), masked_image) plt.figure() plt.imshow(mark_boundaries(img, segments)) plt.contour(roi, contours=1, colors='red', linewidths=0.5) plt.axis('off') plt.savefig(r'I:\18Breakageratecalculation\mask-slic use\maskSLIC-master\result\result6\maskslic.png') #保存图片怎么提取超像素块的位置和大小
时间: 2024-03-22 08:38:08 浏览: 23
要提取超像素块的位置和大小,可以按照以下步骤进行:
1. 遍历所有超像素块,计算每个超像素块的位置和大小信息,例如左上角坐标、宽度和高度等。
2. 将每个超像素块的位置和大小信息保存到一个列表或数组中,以便后续使用。
以下是一个示例代码,使用SLIC算法提取超像素块的位置和大小:
```python
import cv2
import numpy as np
# 加载原始图像
img = cv2.imread('input.jpg')
# 进行SLIC超像素分割
seg = cv2.ximgproc.createSuperpixelSLIC(img, cv2.ximgproc.SLIC, 100, 10)
segments = seg.iterate(10)
# 获取超像素块位置和大小
superpixel_blocks = []
for i in range(seg.getNumberOfSuperpixels()):
mask_i = segments == i
x, y, w, h = cv2.boundingRect(mask_i.astype(np.uint8))
superpixel_blocks.append((x, y, w, h))
# 输出结果
print(superpixel_blocks)
```
请注意,以上代码仅供参考,实际使用时需要根据具体情况进行调整。
相关问题
t1 = time.time() # Note that compactness is defined differently because a grid is not used. Lower compactness for maskSLIC is equivalent segments = seg.slic(img, compactness=10, seed_type='nplace', mask=roi, n_segments=120, recompute_seeds=True, plot_examples=True, enforce_connectivity=True) print("Time: {:.2f} s".format(time.time() - t1)) plt.figure() plt.imshow(mark_boundaries(img, segments)) plt.contour(roi, contours=1, colors='red', linewidths=0.5) plt.axis('off') plt.savefig(r'I:\18Breakageratecalculation\mask-slic use\maskSLIC-master\result\split\result3\maskslic.png') 怎么保存每一块超像素图像 完整代码
完整代码如下:
```
import cv2
import numpy as np
import matplotlib.pyplot as plt
from skimage.segmentation import mark_boundaries
import time
# Load image
img = cv2.imread('image.jpg')
# Create binary mask
h, w = img.shape[:2]
roi = np.zeros((h, w), dtype=np.uint8)
cv2.rectangle(roi, (100, 100), (w-100, h-100), 255, -1)
# Apply maskSLIC
t1 = time.time()
segments = seg.slic(img, compactness=10, seed_type='nplace', mask=roi, n_segments=120, recompute_seeds=True, plot_examples=True, enforce_connectivity=True)
print("Time: {:.2f} s".format(time.time() - t1))
# Save each segment as an individual file
for segment_id in np.unique(segments):
mask = segments == segment_id
masked_image = img.copy()
masked_image[~mask] = 0
cv2.imwrite('segment_{}.png'.format(segment_id), masked_image)
# Show result
plt.figure()
plt.imshow(mark_boundaries(img, segments))
plt.contour(roi, contours=1, colors='red', linewidths=0.5)
plt.axis('off')
plt.savefig(r'I:\18Breakageratecalculation\mask-slic use\maskSLIC-master\result\split\result3\maskslic.png')
```
请注意,此代码使用了一些未定义的变量,例如 `seg`。在使用此代码之前,请确保已经正确导入和定义了这些变量。
img = imread(r'I:\\18Breakageratecalculation\\mask-slic use\\maskSLIC-master\\1\\056.jpg') # The ROI is also stored as an image for viewing convenience # But the roi input input maskSLIC should be a binary image with the same spatial # Dimensions as the image (in this case 300x451) roi = imread(r'I:\\18Breakageratecalculation\\mask-slic use\\maskSLIC-master\\1\\0562.png') # The alpha channel is used to store the ROI in this case and is converted into a logical array of 0s and 1s roi = roi[:, :, 3] > 0 # Alternatively a mask could be created manually with for example a disk: # roi = np.zeros((img.shape[0], img.shape[1])) # a, b = 150, 150 # r = 100 # y,x = np.ogrid[-a:img.shape[0]-a, -b:img.shape[1]-b] # mask = x*x + y*y <= r*r # roi[mask] = 1 # ~~~~~~~~~~~~ Example 1: maskSLIC ~~~~~~~~~~~~~ t1 = time.time() # Note that compactness is defined differently because a grid is not used. Lower compactness for maskSLIC is equivalent segments = seg.slic(img, compactness=10, seed_type='nplace', mask=roi, n_segments=120, recompute_seeds=True, plot_examples=True, enforce_connectivity=True) print("Time: {:.2f} s".format(time.time() - t1)) plt.figure() plt.imshow(mark_boundaries(img, segments)) plt.contour(roi, contours=1, colors='red', linewidths=0.5) plt.axis('off') # ~~~~~~~~~~~ Example 2: SLIC ~~~~~~~~~~~~~~~~~ t1 = time.time() segments = seg.slic(img, compactness=10, seed_type='grid', n_segments=100, plot_examples=False, enforce_connectivity=True) # segments[roi==0] = -1 print("Time: {:.2f} s".format(time.time() - t1)) plt.figure() plt.imshow(mark_boundaries(img, segments)) plt.contour(roi, contours=1, colors='red', linewidths=1) plt.axis('off') plt.show() plt.show()怎么保存结果
如果想要保存分割结果,可以使用matplotlib库中的imsave函数来保存图像。
例如,可以在代码中添加以下语句来保存Example 1中的分割结果:
```
plt.imshow(mark_boundaries(img, segments))
plt.contour(roi, contours=1, colors='red', linewidths=0.5)
plt.axis('off')
plt.savefig('example1.png') # 保存图片
plt.show()
```
这样就会在当前路径下生成一个名为example1.png的图片文件,其中包含分割结果和ROI边界。需要保存Example 2的分割结果时,也可以类似地添加保存语句。
相关推荐
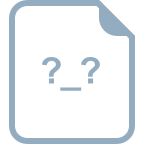
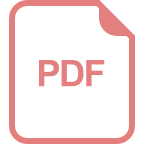
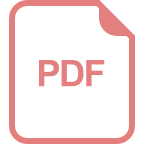






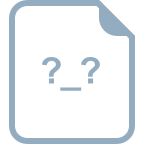
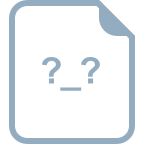
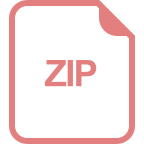