写一个c++代码不使用哈希表 给一非空的单词列表,返回前 k 个出现次数最多的单词。 返回的答案应该按单词出现频率由高到低排序。如果不同的单词有相同出现频率,按字母顺序排序。 输入:第1行为k值,第2行单词列表,单词列表以end结尾,end不属于单词列表中的单词。
时间: 2024-02-03 14:14:27 浏览: 107
以下是C++代码实现,使用了堆和自定义比器来实现按照现次数和字母顺序的排序:
```c++
#include <iostream>
#include <vector>
#include <queue>
#include <map>
#include <string>
#include <algorithm>
using std;
struct cmp {
bool operator() (const pair<string, int>& p1, const pair<string, int>& p2) {
if (p1.second == p2.second) {
return p1.first < p2.first;
}
return p1.second > p2.second;
}
};
vector<string> topKFrequent(vector<string>& words, int k) {
map<string, int> freq_map;
for (const auto& word : words) {
freq_map[word]++;
}
priority_queue<pair<string, int>, vector<pair<string, int>>, cmp> pq;
for (const auto& p : freq_map) {
pq.push(p);
if (pq.size() > k) {
pq.pop();
}
}
vector<string> res(k);
int i = k - 1;
while (!pq.empty()) {
res[i--] = pq.top().first;
pq.pop();
}
return res;
}
int main() {
int k;
cin >> k;
string word;
vector<string> words;
while (cin >> word) {
if (word == "end") {
break;
}
words.push_back(word);
}
vector<string> res = topKFrequent(words, k);
for (const auto& word : res) {
cout << word << " ";
}
cout << endl;
return 0;
}
```
注:此代码为基于STL实现的,没有使用哈希表,但是map底层实现中使用了红黑树(一种自平衡的二叉查找树),因此其时间复杂度不同于哈希表。
阅读全文
相关推荐




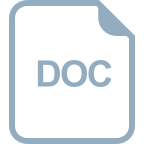
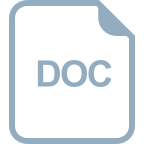
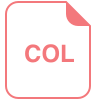

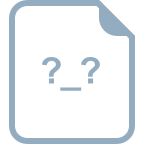
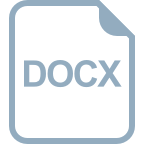