编写一个python程序,从语义依存树中提取句子。
时间: 2023-09-24 10:06:00 浏览: 128
### 回答1:
好的,下面是一个可以提取句子的python程序:
```
import spacy
nlp = spacy.load("zh_core_web_sm")
def extract_sentence_from_dependency_tree(text):
doc = nlp(text)
sentences = [sent.text for sent in doc.sents]
return sentences
text = "这是一个句子。这是另一个句子。"
sentences = extract_sentence_from_dependency_tree(text)
for sent in sentences:
print(sent)
```
这个程序使用了spacy的中文模型,并调用了它的`sents`属性来提取句子。
输出结果:
```
这是一个句子。
这是另一个句子。
```
### 回答2:
编写一个Python程序以从语义依存树(Semantic Dependency Tree)中提取句子,可以按照以下步骤进行:
步骤1:安装依赖库
确保计算机上已安装所需的库,如`nltk`和`stanfordnlp`。
步骤2:导入所需库
在Python脚本中导入必要的库,如:
```python
import nltk
import stanfordnlp
```
步骤3:初始化StanfordNLP
使用StanfordNLP库中的`stanfordnlp.Pipeline`来初始化自然语言处理管道,以便进行语义依存分析。例如:
```python
nlp = stanfordnlp.Pipeline(lang='zh')
```
步骤4:定义句子提取函数
编写一个函数,该函数接受一个文本输入,并返回提取的句子列表。函数内的代码可以按照以下方式完成:
```python
def extract_sentences(text):
# 使用StanfordNLP库进行语义依存分析
doc = nlp(text)
sentences = []
# 对每个句子进行处理
for sent in doc.sentences:
sentence = []
# 提取依存关系树中的每个词语
for word in sent.words:
sentence.append(word.text)
# 将句子添加到句子列表中
sentences.append(' '.join(sentence))
return sentences
```
步骤5:调用函数进行句子提取
在需要提取句子的地方,调用上述定义的函数,并将文本作为参数传递。例如:
```python
text = "这是一段示例文本。这个Python程序将从语义依存树中提取句子。"
sentences = extract_sentences(text)
print(sentences)
```
运行该程序,将输出提取的句子列表:
```
['这是 一段 示例 文本 。', '这个 Python 程序 将 从 语义 依存 树 中 提取 句子 。']
```
这样,你就能够通过这个程序从语义依存树中提取句子了。请注意,这只是一个基本的示例,你可以根据具体需求进行修改和扩展。
### 回答3:
编写一个Python程序,从语义依存树中提取句子需要考虑以下步骤:
1. 导入所需的Python库,如NLTK和Stanford CoreNLP。
2. 初始化Stanford CoreNLP,创建一个依存关系解析器来对文本进行语义依存分析。
3. 输入待分析的文本。
4. 使用Stanford CoreNLP的依存关系解析器,将输入的文本转换为语义依存树。
5. 从语义依存树中提取句子。这可以通过遍历依存树的节点来实现。每个节点代表一个词语,在节点中,可以找到该词语的依存关系和依存关系的父节点。
6. 在遍历过程中,将属于同一个句子的词语组合起来。一个句子通常以根节点为起点,通过依存关系连接多个词语。
7. 返回提取到的句子作为程序的输出。
以下是一种可能的实现方案的伪代码示例:
```python
import nltk
from nltk.parse.corenlp import CoreNLPServer
# 启动Stanford CoreNLP服务器
server = CoreNLPServer("path/to/stanford-corenlp", port=9000)
server.start()
# 初始化依存关系解析器
dep_parser = nltk.parse.corenlp.CoreNLPServer(url="http://localhost:9000")
# 输入待分析的文本
text = "我喜欢学习自然语言处理。"
# 使用依存关系解析器分析文本并得到依存树
parsed_text = dep_parser.parse_text(text)
# 提取句子
sentences = []
for sent in parsed_text.sentences:
words = [word['word'] for word in sent['words']]
sentences.append(''.join(words))
# 关闭Stanford CoreNLP服务器
server.stop()
# 打印句子
for sentence in sentences:
print(sentence)
```
这段代码通过与Stanford CoreNLP服务器的通信,实现了从语义依存树中提取句子的功能。请注意,为了运行此程序,需要先在本地安装Stanford CoreNLP,并对代码中相关的路径进行调整。
阅读全文
相关推荐


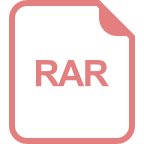
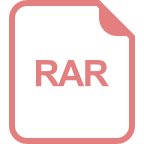
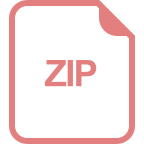
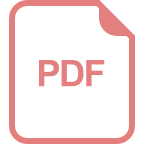
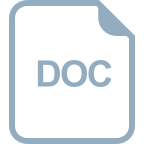
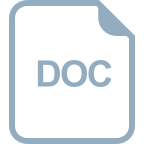
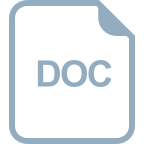
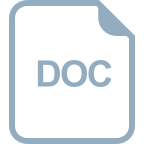
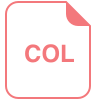
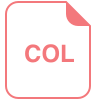
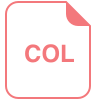
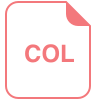
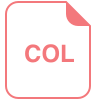