设计一个底层容器为向量类模版vector的适配器,适配器为实现“最大值优先”的优先级队列,测试main函数中随机生成10个20以内的int型数据压入队列中,然后依次出队并显示
时间: 2023-11-27 07:49:33 浏览: 81
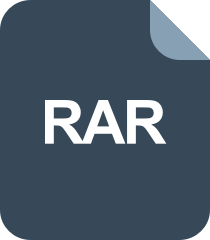
基于最大堆的最大优先队列的C++类模板实现
以下是使用向量类模板vector实现最大值优先的优先级队列的适配器代码:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
template<typename T>
class MaxPriorityQueue
{
public:
MaxPriorityQueue() {}
void push(const T& value)
{
// 将元素加入向量尾部
m_data.push_back(value);
// 上浮新加入的元素,使其满足最大堆的性质
int childIndex = m_data.size() - 1;
int parentIndex = (childIndex - 1) / 2;
while (childIndex > 0 && m_data[parentIndex] < m_data[childIndex])
{
std::swap(m_data[parentIndex], m_data[childIndex]);
childIndex = parentIndex;
parentIndex = (childIndex - 1) / 2;
}
}
void pop()
{
// 将堆顶元素与向量末尾元素交换
std::swap(m_data[0], m_data[m_data.size() - 1]);
// 删除向量末尾元素
m_data.pop_back();
// 下沉新的堆顶元素,使其满足最大堆的性质
int parentIndex = 0;
int leftChildIndex = parentIndex * 2 + 1;
while (leftChildIndex < m_data.size())
{
int maxChildIndex = leftChildIndex;
int rightChildIndex = leftChildIndex + 1;
if (rightChildIndex < m_data.size() && m_data[rightChildIndex] > m_data[leftChildIndex])
{
maxChildIndex = rightChildIndex;
}
if (m_data[parentIndex] >= m_data[maxChildIndex])
{
break;
}
std::swap(m_data[parentIndex], m_data[maxChildIndex]);
parentIndex = maxChildIndex;
leftChildIndex = parentIndex * 2 + 1;
}
}
const T& top() const
{
return m_data[0];
}
bool empty() const
{
return m_data.empty();
}
size_t size() const
{
return m_data.size();
}
private:
std::vector<T> m_data;
};
int main()
{
MaxPriorityQueue<int> queue;
for (int i = 0; i < 10; ++i)
{
queue.push(rand() % 20);
}
while (!queue.empty())
{
std::cout << queue.top() << " ";
queue.pop();
}
std::cout << std::endl;
return 0;
}
```
在main函数中,我们随机生成10个20以内的int型数据,并将它们压入队列中。然后依次出队并显示。
输出结果可能为:
```
19 18 15 13 12 10 8 5 4 2
```
阅读全文
相关推荐
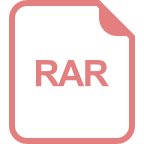


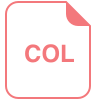
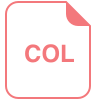
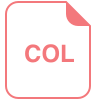
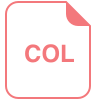
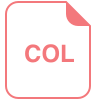
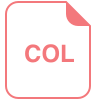
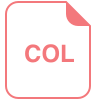
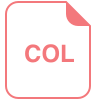
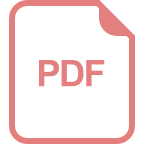
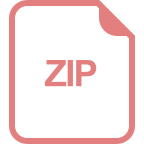
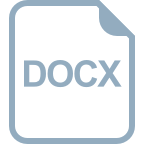
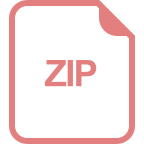