springboot自定义用户登录
时间: 2023-08-29 13:13:23 浏览: 55
在 Spring Boot 中,可以使用 Spring Security 来实现用户认证和授权。下面是一个自定义用户登录的示例:
1. 添加依赖
在 pom.xml 文件中添加 Spring Security 的依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
```
2. 创建用户类
创建一个用户类,用于存储用户信息。可以实现 Spring Security 的 UserDetails 接口,也可以不实现。
```java
public class User {
private String username;
private String password;
// getters and setters
}
```
3. 创建用户服务
创建一个用户服务类,用于提供用户信息。可以从数据库或其他数据源中获取用户信息。
```java
@Service
public class UserService {
public User findByUsername(String username) {
// 从数据库或其他数据源中获取用户信息
return new User(username, "password");
}
}
```
4. 实现 UserDetailsService
实现 Spring Security 的 UserDetailsService 接口,用于从用户服务中获取用户信息,并将其转换为 Spring Security 可以使用的 User 对象。
```java
@Service
public class CustomUserDetailsService implements UserDetailsService {
@Autowired
private UserService userService;
@Override
public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException {
User user = userService.findByUsername(username);
if (user == null) {
throw new UsernameNotFoundException("User not found");
}
return new org.springframework.security.core.userdetails.User(user.getUsername(), user.getPassword(), Collections.emptyList());
}
}
```
5. 配置 Spring Security
在配置类中,配置 Spring Security,包括登录页面、登录请求、登录成功和失败处理等。
```java
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private CustomUserDetailsService userDetailsService;
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/login").permitAll()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.usernameParameter("username")
.passwordParameter("password")
.defaultSuccessUrl("/")
.permitAll()
.and()
.logout()
.permitAll();
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService);
}
}
```
6. 创建登录页面
创建一个登录页面,用于输入用户名和密码。
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Login</title>
</head>
<body>
<h1>Login</h1>
<form action="/login" method="post">
<p>
<label for="username">Username:</label>
<input type="text" id="username" name="username" />
</p>
<p>
<label for="password">Password:</label>
<input type="password" id="password" name="password" />
</p>
<p>
<input type="submit" value="Login" />
</p>
</form>
</body>
</html>
```
以上就是一个简单的 Spring Boot 自定义用户登录的示例。需要注意的是,这里的示例没有使用加密算法对密码进行加密,实际应用中应该对密码进行加密存储。同时,还应该在登录成功后将用户信息存储到 session 或 token 中,以便后续访问需要认证的资源时进行判断。
相关推荐
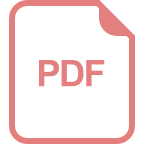
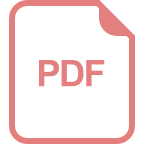
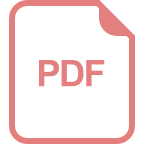














