全景拼接在计算完单应性矩阵后如何进行对齐或平移,请分别给出代码
时间: 2024-05-07 19:21:30 浏览: 93
全景拼接中,对齐或平移是将拼接后的图像进行微调,使得拼接后的图像更加平滑、自然。常用的对齐或平移方法包括基于特征匹配的方法、基于全局优化的方法等。下面分别给出基于特征匹配的对齐和基于全局优化的平移的代码实现。
基于特征匹配的对齐:
```python
import cv2
import numpy as np
# 读取拼接后的图像和单应性矩阵
img = cv2.imread('panorama.jpg')
H = np.loadtxt('H.txt')
# 对拼接后的图像进行对齐
rows, cols = img.shape[:2]
dst = cv2.warpPerspective(img, H, (cols, rows))
# 显示对齐后的图像
cv2.imshow('aligned image', dst)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
其中,`H.txt` 是拼接前计算得到的单应性矩阵文件。
基于全局优化的平移:
```python
import cv2
import numpy as np
# 读取拼接后的图像
img = cv2.imread('panorama.jpg')
# 构建拼接后的图像的掩模
mask = np.zeros(img.shape[:2], dtype=np.uint8)
mask[:, :img.shape[1]//2] = 255
# 定义全局优化函数
def global_optimization(shift, img, mask):
rows, cols = img.shape[:2]
M = np.float32([[1, 0, shift], [0, 1, 0]])
shifted = cv2.warpAffine(img, M, (cols, rows))
return cv2.meanShift(mask, shifted, (0, 0, cols, rows))[0]
# 在x轴方向上进行全局优化
shift, _ = cv2.optimize(global_optimization, 0, (img, mask), method=cv2.OPTPLOT_ANNEALING)
# 对拼接后的图像进行平移
rows, cols = img.shape[:2]
M = np.float32([[1, 0, shift], [0, 1, 0]])
dst = cv2.warpAffine(img, M, (cols, rows))
# 显示平移后的图像
cv2.imshow('shifted image', dst)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
其中,`mask` 是拼接后的图像的掩模,`global_optimization` 函数是全局优化函数,`shift` 是在 x 轴方向上的平移量。这里使用的是 cv2.meanShift 函数进行全局优化。
阅读全文
相关推荐
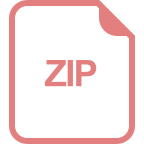


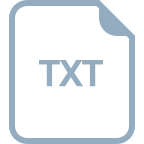
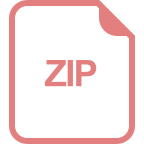
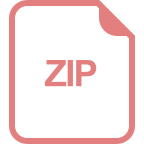
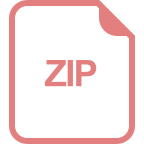
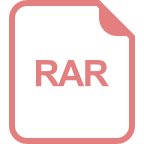
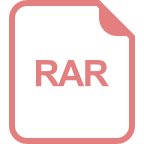
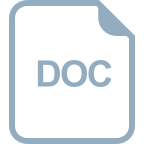
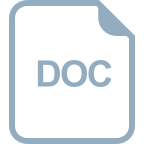
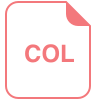
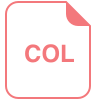
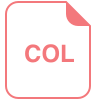
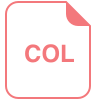
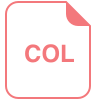