class PartialAlexNet(nn.Module): def __init__(self, # pretrained_path: str = "alex_pretrained/partial.pt"): pretrained_path: str = "partial.pt"): super().__init__() self.net = nn.Sequential( nn.Conv2d(in_channels=3, out_channels=96, kernel_size=(11, 11), stride=(4, 4), padding=(4, 4)), nn.ReLU(inplace=True), nn.LocalResponseNorm(size=5, alpha=0.0001, beta=0.75, k=2.0), nn.MaxPool2d(kernel_size=3, stride=2), nn.Conv2d(in_channels=96, out_channels=256, kernel_size=5, padding=2, groups=2), nn.ReLU(inplace=True), nn.LocalResponseNorm(size=5, alpha=0.0001, beta=0.75, k=2.0), nn.MaxPool2d(kernel_size=3, stride=2) ) self.net.load_state_dict(torch.load(pretrained_path)) 分析该代码
时间: 2023-11-29 08:04:38 浏览: 95
这段代码定义了一个名为 PartialAlexNet 的类,它继承自 nn.Module。该类的初始化方法接收一个参数 pretrained_path,用于指定预训练模型的路径。在初始化方法中,该类定义了一个名为 net 的属性,它是一个由多个卷积层、ReLU激活函数、局部响应归一化层和最大池化层组成的顺序模型。其中,卷积层分别为一个输入通道数为 3,输出通道数为 96,卷积核大小为 (11,11),步幅为 (4,4),填充为 (4,4) 的卷积层,以及一个输入通道数为 96,输出通道数为 256,卷积核大小为 5,填充为 2,组数为 2 的卷积层。ReLU 激活函数和局部响应归一化层分别跟随在每个卷积层之后,而最大池化层则跟随在局部响应归一化层之后。最后,该类使用预训练模型的参数来初始化 net 属性。
相关问题
class Partial_conv3(nn.Module): def __init__(self, dim, n_div, forward): super().__init__() self.dim_conv3 = dim // n_div self.dim_untouched = dim - self.dim_conv3 self.partial_conv3 = nn.Conv2d(self.dim_conv3, self.dim_conv3, 3, 1, 1, bias=False) if forward == 'slicing': self.forward = self.forward_slicing elif forward == 'split_cat': self.forward = self.forward_split_cat else: raise NotImplementedError def forward_slicing(self, x: Tensor) -> Tensor: # only for inference x = x.clone() # !!! Keep the original input intact for the residual connection later x[:, :self.dim_conv3, :, :] = self.partial_conv3(x[:, :self.dim_conv3, :, :]) return x def forward_split_cat(self, x: Tensor) -> Tensor: x1, x2 = torch.split(x, [self.dim_conv3, self.dim_untouched], dim=1) x1 = self.partial_conv3(x1) x = torch.cat((x1, x2), 1) return x 在这段代码中插入全局平均池化做通道增强的模块
可以将全局平均池化放在每个 forward 函数的最后,对每个 channel 进行平均池化操作,然后将结果与未被改变的 channel 拼接起来,得到增强后的输出。修改后的代码如下:
```
class Partial_conv3(nn.Module):
def __init__(self, dim, n_div, forward):
super().__init__()
self.dim_conv3 = dim // n_div
self.dim_untouched = dim - self.dim_conv3
self.partial_conv3 = nn.Conv2d(self.dim_conv3, self.dim_conv3, 3, 1, 1, bias=False)
self.global_avg_pool = nn.AdaptiveAvgPool2d((1, 1)) # 添加全局平均池化层
if forward == 'slicing':
self.forward = self.forward_slicing
elif forward == 'split_cat':
self.forward = self.forward_split_cat
else:
raise NotImplementedError
def forward_slicing(self, x: Tensor) -> Tensor:
# only for inference
x = x.clone() # !!! Keep the original input intact for the residual connection later
x[:, :self.dim_conv3, :, :] = self.partial_conv3(x[:, :self.dim_conv3, :, :])
x[:, :self.dim_conv3, :, :] = self.global_avg_pool(x[:, :self.dim_conv3, :, :]) # 添加全局平均池化
x = torch.cat((x, x[:, self.dim_conv3:, :, :]), 1)
return x
def forward_split_cat(self, x: Tensor) -> Tensor:
x1, x2 = torch.split(x, [self.dim_conv3, self.dim_untouched], dim=1)
x1 = self.partial_conv3(x1)
x1 = self.global_avg_pool(x1) # 添加全局平均池化
x = torch.cat((x1, x2), 1)
return x
```
class MobileNetV2(nn.Module): def __init__(self, downsample_factor=8, pretrained=True): super(MobileNetV2, self).__init__() from functools import partial model = mobilenetv2(pretrained) self.features = model.features[:-1] self.total_idx = len(self.features) self.down_idx = [2, 4, 7, 14] if downsample_factor == 8: for i in range(self.down_idx[-2], self.down_idx[-1]): self.features[i].apply( partial(self._nostride_dilate, dilate=2) ) for i in range(self.down_idx[-1], self.total_idx): self.features[i].apply( partial(self._nostride_dilate, dilate=4) ) elif downsample_factor == 16: for i in range(self.down_idx[-1], self.total_idx): self.features[i].apply( partial(self._nostride_dilate, dilate=2) ) def _nostride_dilate(self, m, dilate): classname = m.__class__.__name__ if classname.find('Conv') != -1: if m.stride == (2, 2): m.stride = (1, 1) if m.kernel_size == (3, 3): m.dilation = (dilate // 2, dilate // 2) m.padding = (dilate // 2, dilate // 2) else: if m.kernel_size == (3, 3): m.dilation = (dilate, dilate) m.padding = (dilate, dilate)
这是一个使用 PyTorch 实现的 MobileNetV2 模型。MobileNetV2 是一种轻量级的卷积神经网络,适用于移动设备和嵌入式设备等资源受限的环境。
该模型的构造函数中有两个参数:downsample_factor 和 pretrained。downsample_factor 控制最后一层卷积的下采样倍数,可以选择 8 或 16。pretrained 控制是否使用预训练的权重。
该模型使用了 MobileNetV2 的预训练模型作为基础模型,然后通过修改其中的一些层来适应不同的下采样倍数。具体来说,它通过将一些卷积层的步幅从 2 改为 1,并在这些层上应用不同的膨胀率来实现下采样倍数的调整。
_nostride_dilate 是一个辅助函数,用于在不改变卷积层大小的情况下增大感受野。它通过在卷积核周围填充一些像素来实现膨胀卷积。
总体来说,这个 MobileNetV2 模型是一个轻量级的卷积神经网络,适用于在资源受限的环境中进行图像分类等任务。
阅读全文
相关推荐
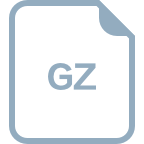
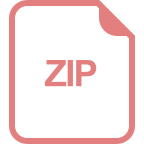


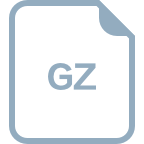
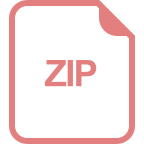
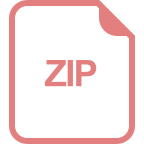
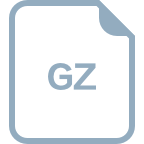
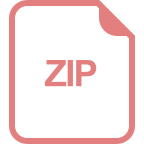
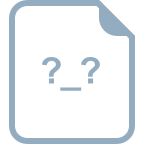
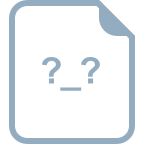
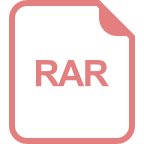
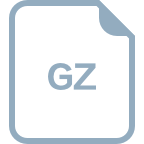
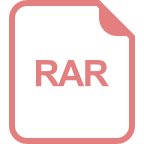
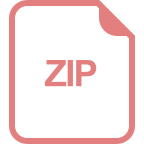