from pyspark import SparkContext from pyspark.sql import SQLContext from pyspark.sql.types import Row from pyspark.sql.types import StructType from pyspark.sql.types import StructField from pyspark.sql.types import StringType from pyspark.sql.types import IntegerType if __name__ == "__main__": sc = SparkContext( 'local', 'test') spark=SQLContext(sc) jdbcDF=spark.read.format("jdbc").option("url","jdbc:mysql://localhost:3306/sparktest").option("driver","com.mysql.jdbc.Driver").option("dbtable","employee").option("user", "root").option("password", "123").load() jdbcDF.filter(jdbcDF.age>20).collect()//检测是否连接成功 studentRDD = sc.parallelize(["3 Mary F 26","4 Tom M 23"]).map(lambda line : line.split(" ")) schema = StructType([StructField("id",IntegerType(),True),StructField("name", StringType(), True),StructField("gender", StringType(), True),StructField("age",IntegerType(), True)]) rowRDD = studentRDD.map(lambda p : Row(int(p[0]),p[1].strip(), p[2].strip(),int(p[3]))) employeeDF = spark.createDataFrame(rowRDD, schema) prop = {} prop['user'] = 'root' prop['password'] = '123' prop['driver'] = "com.mysql.jdbc.Driver" employeeDF.write.jdbc("jdbc:mysql://localhost:3306/sparktest",'employee','append', prop) jdbcDF.collect() jdbcDF.agg({"age": "max"}).show() jdbcDF.agg({"age": "sum"}).show()
时间: 2023-07-19 12:50:59 浏览: 56
这段代码看起来是一个 Spark SQL 的示例代码,它实现了从 MySQL 数据库中读取数据,对数据进行过滤、聚合等操作,然后将结果写回到 MySQL 数据库中。具体来说,代码中使用了以下 Spark SQL API:
1. 通过 `SQLContext` 创建 Spark SQL 的上下文环境
2. 使用 `read.format("jdbc")` 方法从 MySQL 数据库中读取数据
3. 使用 `filter` 方法对数据进行过滤
4. 使用 `collect` 方法将过滤后的数据以 RDD 的形式返回
5. 使用 `createDataFrame` 方法将 RDD 转换为 DataFrame
6. 使用 `write.jdbc` 方法将 DataFrame 中的数据写回到 MySQL 数据库中
7. 使用 `agg` 方法进行聚合操作,例如 `agg({"age": "max"})` 表示计算 age 列的最大值
如果您有具体的问题需要解决,请告诉我。
相关问题
from pyspark import SparkContext from pyspark.sql import SQLContext from pyspark.sql.types import Row from pyspark.sql.types import StructType from pyspark.sql.types import StructField from pyspark.sql.types import StringType from pyspark.sql.types import IntegerType if __name__ == "__main__": sc = SparkContext( 'local', 'test') spark=SQLContext(sc) jdbcDF=spark.read.format("jdbc").option("url","jdbc:mysql://localhost:3306/sparktest").option("driver","com.mysql.jdbc.Driver").option("dbtable","employee").option("user", "root").option("password", "123").load() jdbcDF.filter(jdbcDF.age>20).collect()//检测是否连接成功 studentRDD = sc.parallelize(["3 Mary F 26","4 Tom M 23"]).map(lambda line : line.split(" ")) schema = StructType([StructField("id",IntegerType(),True),StructField("name", StringType(), True),StructField("gender", StringType(), True),StructField("age",IntegerType(), True)]) rowRDD = studentRDD.map(lambda p : Row(int(p[0]),p[1].strip(), p[2].strip(),int(p[3]))) employeeDF = spark.createDataFrame(rowRDD, schema) prop = {} prop['user'] = 'root' prop['password'] = '123' prop['driver'] = "com.mysql.jdbc.Driver" employeeDF.write.jdbc("jdbc:mysql://localhost:3306/sparktest",'employee','append', prop) jdbcDF.collect() jdbcDF.agg({"age": "max"}).show() jdbcDF.agg({"age": "sum"}).show()请给我详细的解释
这段代码是一个使用 PySpark 连接到 MySQL 数据库,读取数据并进行处理,最后将处理结果写回数据库的示例代码。
首先,代码导入了需要的模块:SparkContext、SQLContext、Row,以及处理数据需要用到的数据类型:StructType、StructField、StringType、IntegerType。
然后,通过 SparkContext 创建一个 SparkSession,连接到 MySQL 数据库,读取 employee 表中的数据,并使用 filter 进行筛选,只保留 age 大于 20 的记录。
接下来,代码构造了一个包含 id、name、gender、age 四个字段的数据结构 schema,然后使用 map 函数将一个包含两条记录的 RDD 转换成 Row 类型的 RDD,再使用 createDataFrame 函数将 Row RDD 转换成 DataFrame。
最后,代码将 DataFrame 中的数据写回 MySQL 数据库中的 employee 表中,并对原始的 jdbcDF 进行了两次汇总:计算 age 的最大值和总和,并通过 show 函数展示结果。
pyspark.sql
pyspark.sql是PySpark中用于操作结构化数据的模块。它提供了DataFrame和SQL等多种API,使得PySpark能够像使用关系型数据库一样对数据进行处理。pyspark.sql主要包括以下几个子模块:
1. pyspark.sql.functions:提供了各种用于数据处理和转换的函数,例如聚合函数、字符串处理函数、日期函数等。
2. pyspark.sql.types:定义了DataFrame中各列的数据类型。
3. pyspark.sql.DataFrame:是PySpark中最常用的数据结构,类似于关系型数据库中的表格。
4. pyspark.sql.SQLContext:用于创建DataFrame对象并操作数据,主要支持SQL语言和DataFrame API。
5. pyspark.sql.streaming:用于实现流式数据处理。
在PySpark中,pyspark.sql模块是数据处理的核心,它提供了丰富的API和函数,可以满足各种数据处理需求。
相关推荐
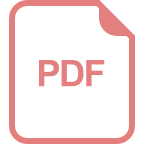
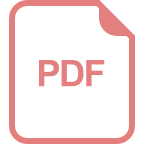
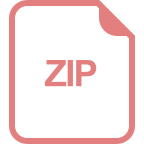













