@GetMapping("/list/{current}")这是什么意思
时间: 2024-03-30 11:36:51 浏览: 21
这是一个 Spring Boot 中的注解,用于处理 HTTP GET 请求。其中的"/list/{current}"是请求的 URL 地址,其中的 "{current}" 是一个路径变量,表示当前页数。在代码中,可以通过 @PathVariable 注解获取这个路径变量的值,然后根据这个值来返回对应的数据。例如,如果当前页数是 1,那么就返回第一页的数据。
相关问题
在下面代码中添加日期和参与比赛总数的接口,日期和参与比赛总数为数据库已有数据: @Api(tags = "Codeforces") @RestController @RequestMapping("/acmer/codeforces") public class CodeforcesController { @Resource private CodeforcesServiceImpl codeforcesService; @ApiOperation("查询全部学生Codeforces信息") @ApiImplicitParams({ @ApiImplicitParam(name = "currentPage",value = "当前页数",required = true), @ApiImplicitParam(name = "pageSize",value = "页面大小",required = true) }) @GetMapping("/all/{currentPage}/{pageSize}") public PublicProperty<Page<Codeforces>> selectAll(@PathVariable("currentPage") Integer currentPage, @PathVariable("pageSize") Integer pageSize){ Page<Codeforces> page = new Page<>(currentPage,pageSize); return new PublicProperty(200,"success",codeforcesService.page(page)); } }
您可以通过以下方式来添加日期和参与比赛总数接口:
1. 首先,从数据库中获取日期和参与比赛总数的数据。您可以使用适当的查询语句从数据库中检索这些数据。
2. 在`Codeforces`实体类中添加日期和参与比赛总数的属性,并生成对应的getter和setter方法。
3. 修改`Codeforces`实体类的`selectAll`方法,将获取到的日期和参与比赛总数数据设置到返回的`Codeforces`对象中。
4. 最后,将修改后的`Codeforces`对象添加到`Page<Codeforces>`中,并将`Page<Codeforces>`对象作为返回值返回。
下面是修改后的代码示例:
```java
@Api(tags = "Codeforces")
@RestController
@RequestMapping("/acmer/codeforces")
public class CodeforcesController {
@Resource
private CodeforcesServiceImpl codeforcesService;
@ApiOperation("查询全部学生Codeforces信息")
@ApiImplicitParams({
@ApiImplicitParam(name = "currentPage",value = "当前页数",required = true),
@ApiImplicitParam(name = "pageSize",value = "页面大小",required = true)
})
@GetMapping("/all/{currentPage}/{pageSize}")
public PublicProperty<Page<Codeforces>> selectAll(@PathVariable("currentPage") Integer currentPage,
@PathVariable("pageSize") Integer pageSize){
Page<Codeforces> page = new Page<>(currentPage, pageSize);
// 从数据库中获取日期和参与比赛总数的数据
List<Codeforces> codeforcesList = codeforcesService.page(page).getRecords();
for (Codeforces codeforces : codeforcesList) {
// 设置日期和参与比赛总数到Codeforces对象中
// 这里假设数据库中的日期字段为date,参与比赛总数字段为participateCount
codeforces.setDate(/*从数据库获取的日期*/);
codeforces.setParticipateCount(/*从数据库获取的参与比赛总数*/);
}
return new PublicProperty(200,"success", codeforcesService.page(page));
}
}
```
请注意,您需要根据您的实际情况修改代码中的注释部分以正确地获取日期和参与比赛总数的数据,并设置到`Codeforces`对象中。
根据 // 获取所有的歌曲列表 @GetMapping("/list") public List<Music> findAllSongs() { return musicService.findAllSongs(); } @GetMapping public Result<Page<Music>> findById(@RequestParam(required = false,value ="1" )Integer pageNum, @RequestParam(required = false,value ="5" )Integer PageSize, @PathVariable(required = false) String name){ musicService.findPage(pageNum,PageSize,name); return Result.success( ); }用uni.request写一个uniapp中调用接口以列表排列,并能够支持分页的前端,端口号为http://localhost:8080/
假设后端API已经实现,并且能够返回正确的数据格式,那么我们可以使用uni.request来发起请求,并使用uni-list来展示数据。下面是一个简单的示例代码:
1. 在页面的data中定义变量:
```javascript
data: {
songs: [], // 存储歌曲列表
pageNum: 1, // 当前页码,默认为1
pageSize: 5, // 每页显示的条数,默认为5
total: 0 // 总记录数
}
```
2. 在页面的onLoad函数中发起请求:
```javascript
onLoad() {
this.loadSongs()
},
methods: {
// 加载歌曲列表
loadSongs() {
uni.request({
url: 'http://localhost:8080/list', // 接口地址
data: {
pageNum: this.pageNum, // 当前页码
pageSize: this.pageSize // 每页显示的条数
},
success: (res) => {
if (res.statusCode === 200) {
const { records, total } = res.data // 从响应数据中获取歌曲列表和总记录数
this.songs = records // 更新歌曲列表
this.total = total // 更新总记录数
}
}
})
}
}
```
3. 使用uni-list来展示歌曲列表:
```html
<template>
<view>
<uni-list>
<uni-list-item v-for="(song, index) in songs" :key="index">
<view slot="title">{{ song.name }}</view>
<view slot="note">{{ song.singer }}</view>
</uni-list-item>
</uni-list>
<uni-pagination :current="pageNum" :total="total" :page-size="pageSize" @change="handlePageChange" />
</view>
</template>
```
4. 在handlePageChange函数中更新页码并重新发起请求:
```javascript
methods: {
// 加载歌曲列表
loadSongs() {
uni.request({
url: 'http://localhost:8080/list', // 接口地址
data: {
pageNum: this.pageNum, // 当前页码
pageSize: this.pageSize // 每页显示的条数
},
success: (res) => {
if (res.statusCode === 200) {
const { records, total } = res.data // 从响应数据中获取歌曲列表和总记录数
this.songs = records // 更新歌曲列表
this.total = total // 更新总记录数
}
}
})
},
// 处理分页器页码变化事件
handlePageChange(pageNum) {
this.pageNum = pageNum // 更新当前页码
this.loadSongs() // 重新加载歌曲列表
}
}
```
相关推荐
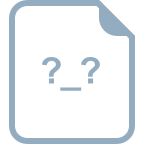
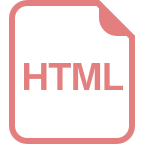













