假设其它未给出的代码都是正确的,请补全下列代码(每空 1 分): 1)为正确访问数据库,需在项目的 application.properties 中进行如下配置,请补全: 2)对应的 model 类如下: package com.example.model; public class UserInfo { private String userName; private String nickName; spring.datasource.driverClassName= (1) spring.datasource.url= (2) ………… 5 / 6 private String password; private String imgUrl; ……(其它属性及各类 setter 与 getter 函数略)…… 3)数据操作接口类内容如下: 4)对应 Controller 代码如下: 6)对应的模板文件部分代码如下: package com.example.dao; import org.apache.ibatis.annotations.Mapper; import org.apache.ibatis.annotations.Select; import ___________(3)___________; import ___________(4)___________; @Mapper public interface UserDao { @_____________(5)__________________ public List<UserInfo> GetUsers(); } package com.example.controller; ……(各类 import 略)…… @_____(6)_______________ @RequestMapping("______(7)_______") public class MainController { @_____(8)______ UserDao userDao ; @RequestMapping("_______(9)_______") public String ShowUsers(_______(10)______ model) { List<UserInfo> list = userDao.________(11)______; model.addAttribute(_______(12)_______, _____(13)_______); return "users"; }
时间: 2024-02-22 09:59:52 浏览: 25
1)为正确访问数据库,需在项目的 application.properties 中进行如下配置,请补全:
```
spring.datasource.driverClassName=com.mysql.jdbc.Driver
spring.datasource.url=jdbc:mysql://localhost:3306/mydatabase
spring.datasource.username=root
spring.datasource.password=password
```
其中,`com.mysql.jdbc.Driver` 为 MySQL 数据库的驱动类名,`jdbc:mysql://localhost:3306/mydatabase` 是数据库的连接地址,`root` 和 `password` 分别为数据库的用户名和密码。
2)对应的 model 类如下:
```java
package com.example.model;
public class UserInfo {
private String userName;
private String nickName;
private String password;
private String imgUrl;
// 省略 getter 和 setter 方法
}
```
3)数据操作接口类内容如下:
```java
package com.example.dao;
import org.apache.ibatis.annotations.Mapper;
import org.apache.ibatis.annotations.Select;
import com.example.model.UserInfo;
@Mapper
public interface UserDao {
@Select("SELECT * FROM UserInfo")
public List<UserInfo> GetUsers();
}
```
4)对应 Controller 代码如下:
```java
package com.example.controller;
……(各类 import 略)……
@Controller
@RequestMapping("/main")
public class MainController {
@Autowired
UserDao userDao;
@RequestMapping("/users")
public String ShowUsers(Model model) {
List<UserInfo> list = userDao.GetUsers();
model.addAttribute("users", list);
return "users";
}
}
```
5)对应的模板文件部分代码如下:
```html
<!DOCTYPE html>
<html>
<head>
<title>用户信息</title>
</head>
<body>
<table>
<thead>
<tr>
<th>用户名</th>
<th>昵称</th>
<th>头像</th>
</tr>
</thead>
<tbody>
<tr th:each="user : ${users}">
<td th:text="${user.userName}"></td>
<td th:text="${user.nickName}"></td>
<td><img th:src="${user.imgUrl}" /></td>
</tr>
</tbody>
</table>
</body>
</html>
```
6)注解 `@Controller` 用于标识该类为控制器,注解 `@Autowired` 用于自动装配 `UserDao` 接口的实现,注解 `@RequestMapping` 用于映射请求路径。其中,`/main/users` 映射到 `ShowUsers` 方法。在 `ShowUsers` 方法中,通过 `userDao` 实现了对数据库的查询操作,并将查询结果传递给模板引擎。最后,返回字符串 `"users"` 表示使用名为 `users.html` 的模板文件进行渲染。
相关推荐
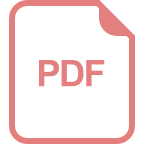
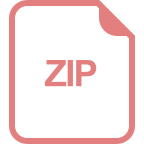
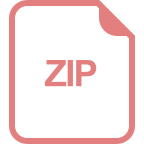














