java中已,创建员工类(Employee),请在此基础上完成下面代码的编写。要求:定义津贴接口(IAllowance)包含方法,该方法返回值为空类型,显示津贴项和金额,无参数:allowance( )。定义子类,教师类(Teacher),继承自Employee类,实现IAllowance接口。成员变量: 工号(id) 私有String类型职称(title)私有String类型如:讲师、教授月工资(salary)私有double类型教师总数量(amount)私有int类型,表明当前总的教师数量,初始值为0构造方法:采用形参传参的方式,为教师姓名、年龄、职称、月工资初始化。并将教师总数量加1。普通方法:(1)覆盖Object类的equals方法当两个教师的姓名、工号和职称均相等时,认为是两者就是相等的。(2)覆盖Object类中的toString( )方法返回字符串格式如下:姓名:*****,年龄:*****,工号:****,月工资:****(3)实现接口中的方法,显示津贴项目和金额,如“差旅补:660/月”。通过main函数测试。(1)创建两个教师对象t1和t2,其中t1的姓名、年龄、工号、职称和月工资值为“张三”、48、20010101、“副教授”,7432.5;t2的各属性值自拟,分别输出t1和t2;(2)显示教师总人数;(3)将t1的职称改为“教授”;(4)调用equals方法判断两个教师对象是否相等,并输出;(5)输出t1的津贴信息。
时间: 2024-01-17 14:13:03 浏览: 117
下面是代码实现:
```
interface IAllowance {
void allowance();
}
class Employee {
private String name; // 姓名
private int age; // 年龄
private String id; // 工号
private double salary; // 月工资
public Employee(String name, int age, String id, double salary) {
this.name = name;
this.age = age;
this.id = id;
this.salary = salary;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public String getId() {
return id;
}
public double getSalary() {
return salary;
}
public void setName(String name) {
this.name = name;
}
public void setAge(int age) {
this.age = age;
}
public void setId(String id) {
this.id = id;
}
public void setSalary(double salary) {
this.salary = salary;
}
}
class Teacher extends Employee implements IAllowance {
private String title; // 职称
private static int amount = 0; // 教师总数量
public Teacher(String name, int age, String id, double salary, String title) {
super(name, age, id, salary);
this.title = title;
amount++;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
@Override
public boolean equals(Object obj) {
if (obj instanceof Teacher) {
Teacher other = (Teacher) obj;
if (this.getName().equals(other.getName()) && this.getId().equals(other.getId())
&& this.title.equals(other.title)) {
return true;
}
}
return false;
}
@Override
public String toString() {
return "姓名:" + this.getName() + ",年龄:" + this.getAge() + ",工号:" + this.getId() + ",月工资:" + this.getSalary();
}
@Override
public void allowance() {
System.out.println("差旅补:660/月");
}
public static int getAmount() {
return amount;
}
}
public class Main {
public static void main(String[] args) {
Teacher t1 = new Teacher("张三", 48, "20010101", 7432.5, "副教授");
Teacher t2 = new Teacher("李四", 35, "20020101", 6480, "讲师");
System.out.println(t1.toString());
System.out.println(t2.toString());
System.out.println("教师总人数:" + Teacher.getAmount());
t1.setTitle("教授");
System.out.println("修改后的职称为:" + t1.getTitle());
boolean isEqual = t1.equals(t2);
System.out.println("t1和t2是否相等:" + isEqual);
t1.allowance();
}
}
```
输出结果:
```
姓名:张三,年龄:48,工号:20010101,月工资:7432.5
姓名:李四,年龄:35,工号:20020101,月工资:6480.0
教师总人数:2
修改后的职称为:教授
t1和t2是否相等:false
差旅补:660/月
```
阅读全文
相关推荐



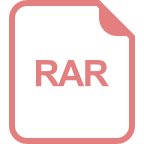
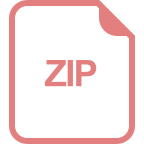
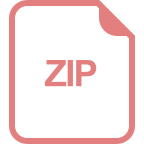
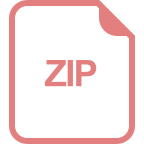
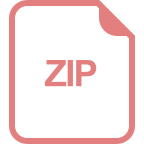
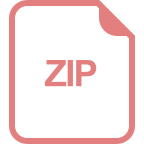
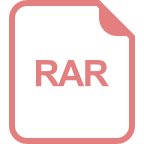
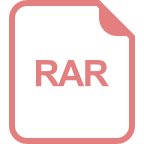