在densenet中添加se模块
时间: 2023-09-25 08:12:27 浏览: 52
在DenseNet中添加SE(Squeeze-and-Excitation)模块可以通过以下步骤实现:
1. 定义SE模块函数:
```
import tensorflow as tf
def se_block(input_tensor, compress_rate=16):
# 获取输入张量的形状
input_shape = input_tensor.get_shape().as_list()
# 计算通道数
num_channels = input_shape[-1]
# 压缩通道数
reduced_channels = num_channels // compress_rate
# Squeeze操作:全局平均池化
squeeze = tf.reduce_mean(input_tensor, [1, 2])
# Excitation操作:全连接层
excitation = tf.layers.dense(squeeze, reduced_channels, activation=tf.nn.relu)
excitation = tf.layers.dense(excitation, num_channels, activation=tf.nn.sigmoid)
# Reshape张量
excitation = tf.reshape(excitation, [-1, 1, 1, num_channels])
# Scale操作:原始张量与Excitation张量相乘
scale = input_tensor * excitation
return scale
```
2. 在DenseBlock中应用SE模块:
```
def dense_block(input_tensor, num_layers, growth_rate, bottleneck_width=4):
# 存储所有密集块中的特征映射
features = [input_tensor]
# 创建num_layers个密集层
for i in range(num_layers):
# 计算Bottleneck层的输出通道数
bottleneck_channels = growth_rate * bottleneck_width
# BN-ReLU-Conv1x1-BN-ReLU-Conv3x3
x = batch_norm_relu(features[-1])
x = conv2d(x, bottleneck_channels, kernel_size=1)
x = batch_norm_relu(x)
x = conv2d(x, growth_rate, kernel_size=3)
# 应用SE模块
x = se_block(x)
# 将当前层的输出添加到特征列表中
features.append(x)
# 将所有特征映射串联在一起
output_tensor = tf.concat(features, axis=-1)
return output_tensor
```
注意:在应用SE模块时,应该添加在密集块的最后一个卷积层之后。
相关推荐
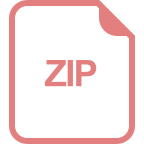
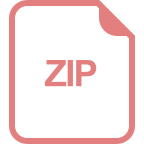
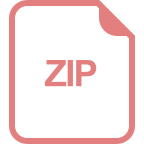








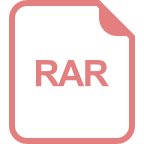
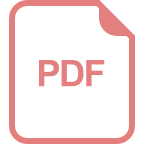
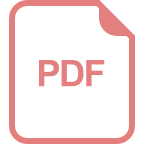