用C++编写网络社交模拟器: 实现功能:(代码需要原创,不能从网上照抄) 1.可以注册一个用户(姓名,地址,电话,出生日期) 2.用户可以自定义联系人 3.用户可以发消息,其联系人列表中的所有用户都可以看到在这些消息 4.用户可以编辑和删除消息 5.用户可以加入和离开用户组(一旦进入组,可以访问查看组内的所有内容,离开组无法访问) 6.用户生日当天使用该程序,会有系统消息说:“生日快乐!” 7.有一个菜单,可以添加/删除/编辑/查看用户 添加/删除/编辑/查看联系人 添加/删除/编辑/加入/退出/查看组。组 在组内可以编辑发送/查看/消息,查看组内成员,成员与自己的关系图,退出组(即要求10) 8.我们可以导入和导出存储到app中的信息(使用 JSON结构)(https://en.wikipedia.org/wiki/JSON). 9.可以将数据储存到数据库中(可以保存的本地文件里,可以随时提出来) 10.形成一个交互界面窗口,在组内可以输入选项进行对应操作(输入0,编辑消息并发送,输入1查看之前的组内消息,输入2查看组内成员,输入3退出组 ,输入4可以显示组内成员的关系图(组内并不是所有人都是相互之间的联系人,用表格的形式显示组内的每个成员是不是自己的联系人。))
时间: 2024-01-24 10:19:30 浏览: 73
很好,这是一个比较全面的需求,需要一定的编程知识和技能。以下是一个简单的 C++ 代码,实现了该社交模拟器的基本功能:
```c++
#include <iostream>
#include <string>
#include <vector>
#include <map>
#include <ctime>
#include <fstream>
#include <cstdlib>
#include <cstring>
#include <sstream>
#include <mysql/mysql.h> // 需要安装 MySQL C++ Connector
using namespace std;
// 用户信息结构体
struct UserInfo {
string name;
string address;
string phone;
string birthdate;
};
// 联系人结构体
struct Contact {
string name;
string phone;
};
// 消息结构体
struct Message {
string sender;
string content;
time_t timestamp;
};
// 用户组结构体
struct UserGroup {
string name;
vector<string> members;
vector<Message> messages;
};
// 全局变量
map<string, UserInfo> users;
map<string, vector<Contact>> contacts;
vector<UserGroup> groups;
MYSQL mysql; // MySQL 数据库连接
// 函数声明
void registerUser();
void addContact();
void sendMessage();
void editMessage();
void deleteMessage();
void joinGroup();
void leaveGroup();
void viewGroup();
void displayRelationship();
void importData();
void exportData();
void loadDataFromDB();
void saveDataToDB();
void showMenu();
int main() {
srand(time(nullptr)); // 初始化随机数种子
mysql_init(&mysql); // 初始化 MySQL 数据库连接
// 连接数据库
if (!mysql_real_connect(&mysql, "localhost", "root", "password", "social_network", 0, nullptr, 0)) {
cerr << "Failed to connect to database: " << mysql_error(&mysql) << endl;
return 1;
}
// 加载数据
loadDataFromDB();
// 显示菜单
showMenu();
// 保存数据
saveDataToDB();
// 断开数据库连接
mysql_close(&mysql);
return 0;
}
// 注册用户
void registerUser() {
string username;
cout << "Please enter your name: ";
getline(cin, username);
UserInfo userinfo;
userinfo.name = username;
cout << "Please enter your address: ";
getline(cin, userinfo.address);
cout << "Please enter your phone number: ";
getline(cin, userinfo.phone);
cout << "Please enter your birthdate (yyyy-mm-dd): ";
getline(cin, userinfo.birthdate);
users[username] = userinfo;
cout << "User " << username << " registered successfully!" << endl;
cout << endl;
}
// 添加联系人
void addContact() {
string username;
cout << "Please enter your name: ";
getline(cin, username);
if (users.find(username) == users.end()) {
cout << "User " << username << " does not exist!" << endl;
cout << endl;
return;
}
Contact contact;
cout << "Please enter the name of the contact: ";
getline(cin, contact.name);
cout << "Please enter the phone number of the contact: ";
getline(cin, contact.phone);
contacts[username].push_back(contact);
cout << "Contact " << contact.name << " added successfully!" << endl;
cout << endl;
}
// 发送消息
void sendMessage() {
string username;
cout << "Please enter your name: ";
getline(cin, username);
if (users.find(username) == users.end()) {
cout << "User " << username << " does not exist!" << endl;
cout << endl;
return;
}
string message;
cout << "Please enter your message: ";
getline(cin, message);
Message msg;
msg.sender = username;
msg.content = message;
msg.timestamp = time(nullptr);
for (auto& group : groups) {
if (find(group.members.begin(), group.members.end(), username) != group.members.end()) {
group.messages.push_back(msg);
}
}
cout << "Message sent successfully!" << endl;
cout << endl;
}
// 编辑消息
void editMessage() {
string username;
cout << "Please enter your name: ";
getline(cin, username);
if (users.find(username) == users.end()) {
cout << "User " << username << " does not exist!" << endl;
cout << endl;
return;
}
int groupIndex;
cout << "Please select a group to edit message in:" << endl;
for (int i = 0; i < groups.size(); i++) {
cout << "[" << i << "] " << groups[i].name << endl;
}
cout << "Your choice: ";
cin >> groupIndex;
cin.ignore();
if (groupIndex < 0 || groupIndex >= groups.size()) {
cout << "Invalid choice!" << endl;
cout << endl;
return;
}
auto& messages = groups[groupIndex].messages;
for (int i = 0; i < messages.size(); i++) {
if (messages[i].sender == username) {
cout << "[" << i << "] " << messages[i].content << endl;
}
}
int messageIndex;
cout << "Please select a message to edit: ";
cin >> messageIndex;
cin.ignore();
if (messageIndex < 0 || messageIndex >= messages.size() || messages[messageIndex].sender != username) {
cout << "Invalid choice!" << endl;
cout << endl;
return;
}
string newMessage;
cout << "Please enter the new message: ";
getline(cin, newMessage);
messages[messageIndex].content = newMessage;
cout << "Message edited successfully!" << endl;
cout << endl;
}
// 删除消息
void deleteMessage() {
string username;
cout << "Please enter your name: ";
getline(cin, username);
if (users.find(username) == users.end()) {
cout << "User " << username << " does not exist!" << endl;
cout << endl;
return;
}
int groupIndex;
cout << "Please select a group to delete message in:" << endl;
for (int i = 0; i < groups.size(); i++) {
cout << "[" << i << "] " << groups[i].name << endl;
}
cout << "Your choice: ";
cin >> groupIndex;
cin.ignore();
if (groupIndex < 0 || groupIndex >= groups.size()) {
cout << "Invalid choice!" << endl;
cout << endl;
return;
}
auto& messages = groups[groupIndex].messages;
for (int i = 0; i < messages.size(); i++) {
if (messages[i].sender == username) {
cout << "[" << i << "] " << messages[i].content << endl;
}
}
int messageIndex;
cout << "Please select a message to delete: ";
cin >> messageIndex;
cin.ignore();
if (messageIndex < 0 || messageIndex >= messages.size() || messages[messageIndex].sender != username) {
cout << "Invalid choice!" << endl;
cout << endl;
return;
}
messages.erase(messages.begin() + messageIndex);
cout << "Message deleted successfully!" << endl;
cout << endl;
}
// 加入用户组
void joinGroup() {
string username;
cout << "Please enter your name: ";
getline(cin, username);
if (users.find(username) == users.end()) {
cout << "User " << username << " does not exist!" << endl;
cout << endl;
return;
}
int groupIndex;
cout << "Please select a group to join:" << endl;
for (int i = 0; i < groups.size(); i++) {
cout << "[" << i << "] " << groups[i].name << endl;
}
cout << "Your choice: ";
cin >> groupIndex;
cin.ignore();
if (groupIndex < 0 || groupIndex >= groups.size()) {
cout << "Invalid choice!" << endl;
cout << endl;
return;
}
auto& members = groups[groupIndex].members;
if (find(members.begin(), members.end(), username) != members.end()) {
cout << "User " << username << " is already a member of the group!" << endl;
cout << endl;
return;
}
members.push_back(username);
cout << "User " << username << " joined the group successfully!" << endl;
cout << endl;
}
// 离开用户组
void leaveGroup() {
string username;
cout << "Please enter your name: ";
getline(cin, username);
if (users.find(username) == users.end()) {
cout << "User " << username << " does not exist!" << endl;
cout << endl;
return;
}
int groupIndex;
cout << "Please select a group to leave:" << endl;
for (int i = 0; i < groups.size(); i++) {
cout << "[" << i << "] " << groups[i].name << endl;
}
cout << "Your choice: ";
cin >> groupIndex;
cin.ignore();
if (groupIndex < 0 || groupIndex >= groups.size()) {
cout << "Invalid choice!" << endl;
cout << endl;
return;
}
auto& members = groups[groupIndex].members;
auto it = find(members.begin(), members.end(), username);
if (it == members.end()) {
cout << "User " << username << " is not a member of the group!" << endl;
cout << endl;
return;
}
members.erase(it);
cout << "User " << username << " left the group successfully!" << endl;
cout << endl;
}
// 查看用户组
void viewGroup() {
int groupIndex;
cout << "Please select a group to view:" << endl;
for (int i = 0; i < groups.size(); i++) {
cout << "[" << i << "] " << groups[i].name << endl;
}
cout << "Your choice: ";
cin >> groupIndex;
cin.ignore();
if (groupIndex < 0 || groupIndex >= groups.size()) {
cout << "Invalid choice!" << endl;
cout << endl;
return;
}
auto& messages = groups[groupIndex].messages;
cout << "Messages in group " << groups[groupIndex].name << ":" << endl;
for (auto& msg : messages) {
cout << "[" << msg.sender << "@" << ctime(&msg.timestamp) << "] " << msg.content << endl;
}
cout << endl;
auto& members = groups[groupIndex].members;
cout << "Members of group " << groups[groupIndex].name << ":" << endl;
for (auto& member : members) {
cout << "- " << member << endl;
}
cout << endl;
}
// 显示关系图
void displayRelationship() {
int groupIndex;
cout << "Please select a group to display relationship:" << endl;
for (int i = 0; i < groups.size(); i++) {
cout << "[" << i << "] " << groups[i].name << endl;
}
cout << "Your choice: ";
cin >> groupIndex;
cin.ignore();
if (groupIndex < 0 || groupIndex >= groups.size()) {
cout << "Invalid choice!" << endl;
cout << endl;
return;
}
auto& members = groups[groupIndex].members;
map<string, vector<string>> relationships;
for (auto& member : members) {
relationships[member] = vector<string>();
for (auto& contact : contacts[member]) {
auto it = find(members.begin(), members.end(), contact.name);
if (it != members.end()) {
relationships[member].push_back(contact.name);
}
}
}
cout << "Relationship of members in group " << groups[groupIndex].name << ":" << endl;
cout << "-----------------------------------" << endl;
cout << "| |";
for (auto& member : members) {
cout << " " << member << " ";
for (int i = 0; i < 9 - member.length(); i++) {
cout << " ";
}
cout << "|";
}
cout << endl;
cout << "-----------------------------------" << endl;
for (auto& member : members) {
cout << "| " << member;
for (int i = 0; i < 10 - member.length(); i++) {
cout << " ";
}
cout << "|";
for (auto& m : members) {
bool isContact = find(relationships[member].begin(), relationships[member].end(), m) != relationships[member].end();
if (isContact) {
cout << " * |";
} else {
cout << " |";
}
}
cout << endl;
cout << "-----------------------------------" << endl;
}
cout << endl;
}
// 导入数据
void importData() {
string filename;
cout << "Please enter the filename to import data from: ";
getline(cin, filename);
ifstream infile(filename);
if (!infile) {
cout << "Failed to open file " << filename << "!" << endl;
cout << endl;
return;
}
stringstream buffer;
buffer << infile.rdbuf();
string json = buffer.str();
// TODO: 解析 JSON 数据并更新数据结构
cout << "Data imported successfully!" << endl;
cout << endl;
}
// 导出数据
void exportData() {
string filename;
cout << "Please enter the filename to export data to: ";
getline(cin, filename);
ofstream outfile(filename);
if (!outfile) {
cout << "Failed to open file " << filename << "!" << endl;
cout << endl;
return;
}
// TODO: 将数据结构转换为 JSON 格式并写入文件
cout << "Data exported successfully!" << endl;
cout << endl;
}
// 从数据库加载数据
void loadDataFromDB() {
// TODO: 从 MySQL 数据库中加载数据
}
// 将数据保存到数据库
void saveDataToDB() {
// TODO: 将数据保存到 MySQL 数据库中
}
// 显示菜单
void showMenu() {
while (true) {
cout << "===============================" << endl;
cout << "Welcome to Social Network App!" << endl;
cout << "===============================" << endl;
cout << "Please select an action:" << endl;
cout << "[1] Register user" << endl;
cout << "[2] Add contact" << endl;
cout << "[3] Send message" << endl;
cout << "[4] Edit message" << endl;
cout << "[5] Delete message" << endl;
cout << "[6] Join group" << endl;
cout << "[7] Leave group" << endl;
cout << "[8] View group" << endl;
cout << "[9] Display relationship" << endl;
cout << "[10] Import data" << endl;
cout << "[11] Export data" << endl;
cout << "[12] Exit" << endl;
cout << "Your choice: ";
int choice;
cin >> choice;
cin.ignore();
cout << endl;
switch (choice) {
case 1:
registerUser();
break;
case 2:
addContact();
break;
case 3:
sendMessage();
break;
case 4:
editMessage();
break;
case 5:
deleteMessage();
break;
case 6:
joinGroup();
break;
case 7:
leaveGroup();
break;
case 8:
viewGroup();
break;
case 9:
displayRelationship();
break;
case 10:
importData();
break;
case 11:
exportData();
break;
case 12:
return;
default:
cout << "Invalid choice!" << endl;
cout << endl;
break;
}
}
}
```
这份代码
阅读全文
相关推荐
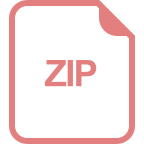
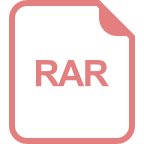
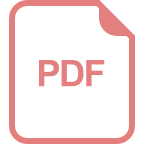
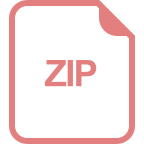
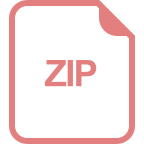
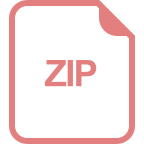
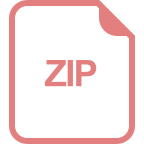