计算用货车,汽车和飞机托运行李的费用java程序
时间: 2024-09-12 16:04:16 浏览: 63
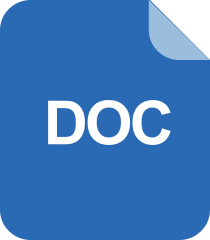
汽车理论作业-Matlab程序-轻型货车动力性能评价.doc
在Java中编写一个计算托运行李费用的程序,通常需要创建一个类来代表交通工具,并包含对应于每种交通工具的行李费用计算逻辑。我们可以假设有不同的价格策略,比如货车、汽车有固定的费用,而飞机可能按重量计费。
这是一个简单的示例:
```java
public class Transportation {
private double baseFee;
private double perKgFee;
// 货车 - 固定费用
public Transportation(String type, double base) {
if ("货车".equals(type)) {
this.baseFee = base;
} else {
this.perKgFee = 0; // 其他交通工具暂设无每公斤费用
}
}
// 汽车 - 同样固定费用
public Transportation(String type, double base) {
if ("汽车".equals(type)) {
this.baseFee = base;
} else {
this.perKgFee = 0;
}
}
// 飞机 - 按重量计费
public Transportation(String type, double base, double perKg) {
if ("飞机".equals(type)) {
this.baseFee = base;
this.perKgFee = perKg;
} else {
throw new IllegalArgumentException("Invalid transportation type");
}
}
public double calculateCharge(double weight) {
if (perKgFee > 0) {
return baseFee + weight * perKgFee;
} else {
return baseFee;
}
}
}
// 使用示例
public class Main {
public static void main(String[] args) {
Transportation truck = new Transportation("货车", 50);
double truckCharge = truck.calculateCharge(200); // 输出200元
Transportation car = new Transportation("汽车", 30);
double carCharge = car.calculateCharge(150); // 输出30元
Transportation plane = new Transportation("飞机", 100, 2); // 每公斤2元
double planeCharge = plane.calculateCharge(500); // 输出600元
}
}
```
阅读全文
相关推荐
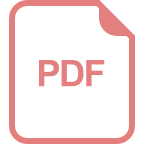

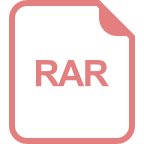
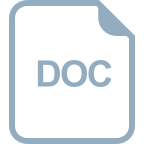
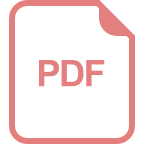
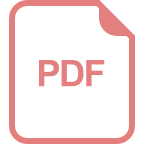
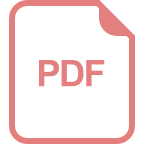
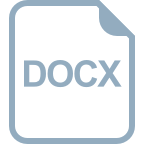
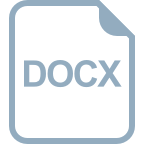
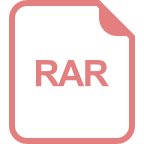
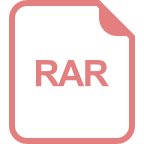
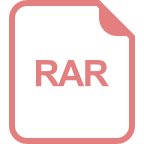

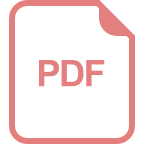
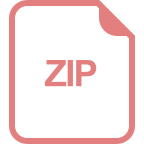