verilog中使用$readmemh对ram初始化
时间: 2024-03-03 08:49:35 浏览: 296
在Verilog中,可以使用$readmemh系统任务对RAM进行初始化。该任务将一个.hex文件读入内存中,并将其用作初始化数据。以下是一个使用$readmemh初始化RAM的示例代码:
```
module ram (
input wire clk,
input wire [7:0] addr,
input wire wr_en,
input wire [7:0] data_in,
output wire [7:0] data_out
);
reg [7:0] mem [255:0];
initial $readmemh("ram_init.hex", mem);
always @(posedge clk) begin
if (wr_en) mem[addr] <= data_in;
data_out <= mem[addr];
end
endmodule
```
在这个例子中,RAM的大小为256个8位字节,地址采用8位宽,数据输入和输出也是8位宽。$readmemh任务将名为"ram_init.hex"的.hex文件读入内存中,并在仿真开始时对RAM进行初始化。在时钟上升沿时,如果wr_en为1,则将data_in写入地址addr处的RAM中;否则从地址addr处读取数据并将其输出到data_out中。
相关问题
verilog初始化ram
在 Verilog 中,可以使用 $readmemh 或者 $readmemb 系统任务来初始化 RAM。这两个任务可以从文件中读取数据并将其写入内存中。
以下是一个使用 $readmemh 的 RAM 初始化的例子:
```
module ram (
input wire [7:0] address,
input wire [7:0] data_in,
input wire write_en,
output reg [7:0] data_out
);
reg [7:0] mem [0:255];
initial begin
$readmemh("ram_init.txt", mem);
end
always @(posedge write_en) begin
mem[address] <= data_in;
end
assign data_out = mem[address];
endmodule
```
在上面的例子中,我们定义了一个 8 位地址和数据的 RAM,以及一个写使能信号和一个数据输出。我们使用 $readmemh 来从一个名为 "ram_init.txt" 的文件中读取数据并初始化 RAM。然后我们使用 always 块来处理写入操作,同时使用 assign 语句来处理读出操作。
module ram_test; // Inputs reg clka; reg [0:0] wea; reg [5:0] addra; reg [31:0] dina; // Outputs wire [31:0] douta; // Instantiate the Unit Under Test (UUT) ram uut ( .clka(clka), .wea(wea), .addra(addra), .dina(dina), .douta(douta) ); reg[63:0]data[0:31]; initial begin // Initialize Inputs clka = 0; wea = 0; addra = 0; dina = 0; end // Wait 100 ns for global reset to finish // 时钟信号 initial begin clka = 0; forever #10 clka = ~clka; end // 写入数据 initial begin wea = 1; addra = 0; repeat (64*4) begin // 从文件中读取数据 $readmemh("data_file.txt", data); // 将数据写入存储器 dina = data[addra % 64]; #10; addra = addra + 1; end wea = 0; end // 读取数据 initial begin addra = 0; repeat (16) begin #10; $display("Data at address %0d: %h", addra, douta); addra = addra + 1; end end endmodulemodule ram_test; // Inputs reg clka; reg [0:0] wea; reg [5:0] addra; reg [31:0] dina; // Outputs wire [31:0] douta; // Instantiate the Unit Under Test (UUT) ram uut ( .clka(clka), .wea(wea), .addra(addra), .dina(dina), .douta(douta) ); reg[63:0]data[0:31]; initial begin // Initialize Inputs clka = 0; wea = 0; addra = 0; dina = 0; end // Wait 100 ns for global reset to finish // 时钟信号 initial begin clka = 0; forever #10 clka = ~clka; end // 写入数据 initial begin wea = 1; addra = 0; repeat (64*4) begin // 从文件中读取数据 $readmemh("data_file.txt", data); // 将数据写入存储器 dina = data[addra % 64]; #10; addra = addra + 1; end wea = 0; end // 读取数据 initial begin addra = 0; repeat (16) begin #10; $display("Data at address %0d: %h", addra, douta); addra = addra + 1; end end endmodule
这段代码是一个简单的 Verilog 代码,用于测试 RAM 存储器模块。代码中包含了 RAM 模块的输入和输出信号,以及存储器的初始化、写入和读取操作。
在初始化过程中,输入信号被初始化为 0,然后通过时钟信号进行重置。然后,通过 `$readmemh` 函数从文件中读取数据,并将数据写入存储器。最后,通过 `$display` 函数输出存储器中的数据。
需要注意的是,代码中使用了 Verilog 的一些语法,例如 `initial` 和 `repeat` 关键字,以及 `$readmemh` 和 `$display` 系统函数。如果您不熟悉 Verilog 语言,建议先学习一下 Verilog 的基本语法。
阅读全文
相关推荐
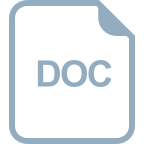
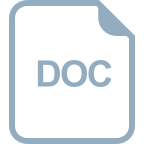
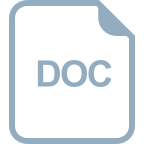
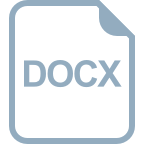
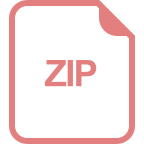
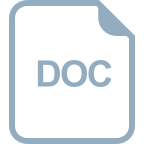
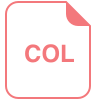


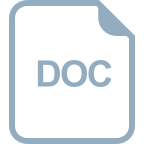
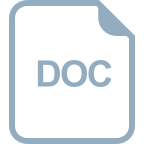