import java.util.Scanner; public class Main { public static void main(String[] args) { int[] num = new int[5]; Scanner in = new Scanner(System.in); String str = in.next(); while(str.equals("other")==false) { try { if(str.equals("null")) { throw new NullPointerException(); } else if(str.equals("arr")) { int i = in.nextInt(); if(i>4||i<0) { throw new ArrayIndexOutOfBoundsException("Index "+i+" out of bounds for length 5"); } } else if(str.equals("cast")) { throw new ClassCastException("class java.lang.String cannot be cast to class java.lang.Integer (java.lang.String and java.lang.Integer are in module java.base of loader 'bootstrap')"); } else if(str.equals("num")) { String str1 = in.next(); if(!(str1.charAt(0)>='0'&&str1.charAt(0)<='9')) { throw new NumberFormatException("For input string: \""+str1+"\""); } } }catch (Exception e) { System.out.println(e); } str = in.next(); } } }
时间: 2023-06-17 12:02:56 浏览: 55
这段代码是一个 Java 程序,主要功能是从控制台读取用户输入的字符串,根据不同的输入内容抛出不同的异常。
具体来说,程序首先创建了一个长度为 5 的整型数组 num,然后通过 Scanner 类从控制台获取用户输入的字符串。接着程序进入一个 while 循环,只要用户输入的字符串不等于 "other",就会根据不同的输入内容抛出不同的异常。
如果用户输入的字符串是 "null",则程序会抛出一个 NullPointerException 异常;如果用户输入的字符串是 "arr",则程序会读取下一个整数 i,如果 i 的值不在数组 num 的有效下标范围内,就会抛出一个 ArrayIndexOutOfBoundsException 异常;如果用户输入的字符串是 "cast",则程序会抛出一个 ClassCastException 异常;如果用户输入的字符串是 "num",则程序会读取下一个字符串 str1,如果 str1 不是一个合法的整数,就会抛出一个 NumberFormatException 异常。
每次程序抛出异常时,会通过 System.out.println() 方法将异常信息打印到控制台。最后,程序会再次等待用户输入,直到用户输入的字符串为 "other",循环结束,程序退出。
相关问题
请仔细阅读下面的代码,根据方法内的提示,在 Begin - End 区域内进行代码补充,具体任务如下: 编辑器中的代码运行时可能会有异常,请利用相关知识处理该异常。 import java.util.Scanner; public class Main { public static void main(String[] args) { Scanner sc = new Scanner(System.in); int num1 = sc.nextInt();
在 Begin - End 区域内进行代码补充,使得程序能够正常运行并输出结果。
```
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int num1 = sc.nextInt();
int num2 = sc.nextInt();
int result = 0;
try {
// Begin
result = num1 / num2;
// End
} catch (ArithmeticException e) {
System.out.println("除数不能为0");
return;
}
System.out.println(result);
}
}
```
import java.util.HashSet; import java.util.Scanner; import java.util.Set; public class Main { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); String input = scanner.nextLine(); String[] numbers = input.split(" "); Set<Integer> set = new HashSet<>(); for (String number : numbers) { int num = Integer.parseInt(number); if (!set.add(num)) { System.out.println("yes"); return; } } System.out.println("no"); }加解释
这段代码实现了一个功能:读入一行输入,将其中的数字以空格分隔开,判断是否存在重复数字。
具体实现步骤:
1. 首先创建一个 Scanner 对象,读取一行输入。
2. 使用 String 类的 split 方法,将输入字符串按照空格分隔成多个字符串,存储在一个字符串数组中。
3. 创建一个 HashSet 对象,用于存储已经出现过的数字。
4. 遍历字符串数组中的每一个字符串,使用 Integer 类的 parseInt 方法将其转换成整数。
5. 判断这个整数是否已经存在于 HashSet 中,如果已经存在,则说明存在重复数字,输出 "yes" 并结束程序。
6. 如果整个字符串数组都遍历完毕,没有发现重复数字,则输出 "no"。
需要注意的是,这里使用 HashSet 来存储已经出现过的数字,是因为 HashSet 具有自动去重的功能,可以方便地判断一个数字是否已经出现过。如果使用其他数据结构,比如数组或者列表,需要手动进行去重操作,会增加代码的复杂度和运行时间。
相关推荐
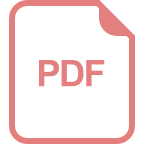
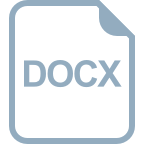
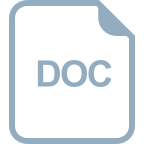







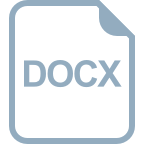
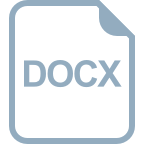
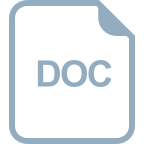
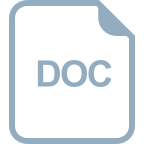
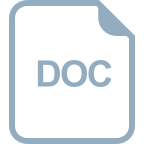
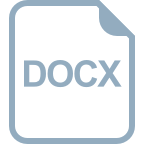
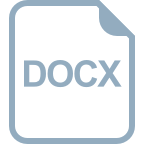
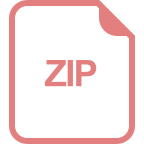