基于mpi4py和multiprocess的并行遗传算法用于解决旅行商问题的python代码
时间: 2024-05-08 11:21:35 浏览: 11
以下是基于mpi4py和multiprocess的并行遗传算法解决旅行商问题的Python代码。代码实现了基本的遗传算法框架和旅行商问题的适应度函数。你可以根据需要进行修改和优化。
```
import random
import numpy as np
from mpi4py import MPI
from multiprocessing import Pool
# MPI初始化
comm = MPI.COMM_WORLD
rank = comm.Get_rank()
size = comm.Get_size()
# 旅行商问题的城市坐标
CITIES = [(60, 200), (180, 200), (80, 180), (140, 180), (20, 160), (100, 160), (200, 160), (140, 140), (40, 120), (100, 120), (180, 100), (60, 80), (120, 80), (180, 60), (20, 40), (100, 40), (200, 40), (20, 20), (60, 20), (160, 20)]
# 旅行商问题的适应度函数
def fitness(solution):
distance = 0
for i in range(len(solution) - 1):
distance += np.sqrt((CITIES[solution[i]][0] - CITIES[solution[i+1]][0])**2 + (CITIES[solution[i]][1] - CITIES[solution[i+1]][1])**2)
distance += np.sqrt((CITIES[solution[-1]][0] - CITIES[solution[0]][0])**2 + (CITIES[solution[-1]][1] - CITIES[solution[0]][1])**2)
return 1 / distance
# 遗传算法的基本框架
def genetic_algorithm(population_size, num_generations, crossover_rate, mutation_rate):
# 创建初始种群
population = []
for i in range(population_size):
individual = list(range(len(CITIES)))
random.shuffle(individual)
population.append(individual)
for generation in range(num_generations):
# 计算适应度
fitness_scores = [fitness(individual) for individual in population]
# 选择
selected_indices = np.random.choice(population_size, size=population_size, replace=True, p=fitness_scores/np.sum(fitness_scores))
selected_population = [population[i] for i in selected_indices]
# 交叉
for i in range(0, population_size, 2):
if random.uniform(0, 1) < crossover_rate:
cross_point = random.randint(1, len(CITIES) - 1)
selected_population[i][cross_point:], selected_population[i+1][cross_point:] = selected_population[i+1][cross_point:], selected_population[i][cross_point:]
# 变异
for i in range(population_size):
if random.uniform(0, 1) < mutation_rate:
mutation_indices = random.sample(range(len(CITIES)), 2)
selected_population[i][mutation_indices[0]], selected_population[i][mutation_indices[1]] = selected_population[i][mutation_indices[1]], selected_population[i][mutation_indices[0]]
# 合并种群
population = selected_population
# 返回最优个体
return max(population, key=fitness)
if __name__ == '__main__':
# 设置参数
population_size = 1000
num_generations = 100
crossover_rate = 0.8
mutation_rate = 0.2
# 并行化遗传算法
if rank == 0:
# 主进程将种群分发给其他进程
pool = Pool(processes=size)
population = [pool.apply_async(genetic_algorithm, args=(population_size//size, num_generations, crossover_rate, mutation_rate)) for i in range(size)]
population = [p.get() for p in population]
population = [y for x in population for y in x]
pool.close()
pool.join()
# 汇总最优解
best_individual = max(population, key=fitness)
best_fitness = fitness(best_individual)
for i in range(1, size):
comm.send(best_fitness, dest=i, tag=0)
comm.send(best_individual, dest=i, tag=1)
else:
# 其他进程执行遗传算法
population = [genetic_algorithm(population_size//size, num_generations, crossover_rate, mutation_rate) for i in range(size)]
best_fitness = comm.recv(source=0, tag=0)
best_individual = comm.recv(source=0, tag=1)
if fitness(population[rank]) > best_fitness:
best_individual = population[rank]
best_fitness = fitness(best_individual)
# 输出结果
if rank == 0:
print("Best individual:", best_individual)
print("Best fitness:", best_fitness)
```
相关推荐
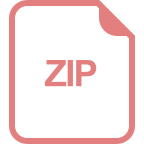
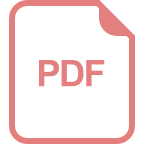
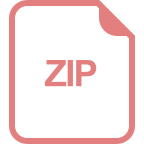














