c# MongoDB Date与本地时间不同
时间: 2024-01-05 19:01:57 浏览: 143
在使用C#操作MongoDB时,如果您在存储日期时遇到本地时间不同的问题,可能是因为MongoDB默认使用UTC时间,而C#默认使用本地时间。这可能会导致存储的日期与您期望的不同。
为了解决这个问题,您可以在存储日期之前将其转换为UTC时间,以确保正确的存储和检索。您可以使用以下代码将本地时间转换为UTC时间:
```
DateTime localTime = DateTime.Now;
DateTime utcTime = localTime.ToUniversalTime();
```
同样,在检索日期时,您需要将存储在MongoDB中的UTC时间转换回本地时间。您可以使用以下代码将UTC时间转换为本地时间:
```
DateTime utcTime = DateTime.UtcNow;
DateTime localTime = utcTime.ToLocalTime();
```
通过这种方式,您可以确保MongoDB和C#之间的日期和时间的一致性,并避免在存储和检索日期时出现问题。
相关问题
C#MONGODB求和
在C#中使用MongoDB进行求和操作可以通过使用聚合管道来实现。下面是一个示例代码,展示了如何使用C#驱动程序进行求和操作:
```csharp
var result = collection.Aggregate()
.Group(x => 1, g => new { Total = g.Sum(x => x.SomeNumberField) })
.FirstOrDefault();
if (result != null)
{
Console.WriteLine(result.Total);
}
```
在这个示例中,我们使用`Aggregate()`方法创建了一个聚合管道。然后,我们使用`Group()`方法将所有文档分组到一个组中,并使用`Sum()`方法对`SomeNumberField`字段进行求和操作。最后,我们使用`FirstOrDefault()`方法获取第一个分组结果,并打印出求和的结果。
请注意,这只是一个示例代码,你需要根据你的具体需求进行适当的修改。同时,确保你已经正确地设置了MongoDB连接和集合的引用。
引用:
\[3\] 进行分组,您可以如下执行: var result = collection.Aggregate() .Group( x => x.SomeStringField, g => new { Result = g.Select( x => x.SomeNumberField ).Max() } ).ToList(); result.ForEach(doc => Console.WriteLine(doc.ToJson()));
#### 引用[.reference_title]
- *1* *2* [MongoDB C# Driver--Date Time](https://blog.csdn.net/qq_44713858/article/details/125788696)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^control_2,239^v3^insert_chatgpt"}} ] [.reference_item]
- *3* [C# MongoDB Aggregate 分组统计](https://blog.csdn.net/yuanpan/article/details/123380676)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^control_2,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
C# 连接mongo 将标准时间区间转为10位时间戳区间并查询
在C#中连接MongoDB,你需要使用MongoDB的官方驱动程序MongoDB.Driver,首先安装它,然后可以创建一个时间区间转换为10位时间戳范围的函数,用于查询特定时间段的数据。以下是基本步骤:
1. 安装MongoDB.NET驱动:
```sh
dotnet add package MongoDB.Driver
```
2. 创建连接和数据库实例:
```csharp
var mongoClient = new MongoClient("mongodb://localhost:27017");
var database = mongoClient.GetDatabase("your_database_name");
var collection = database.GetCollection<BsonDocument>("your_collection_name");
```
3. 定义一个辅助函数,将`BsonDate`类型的时间转换为10位时间戳:
```csharp
private static long DateToTimestamp(BsonDate date)
{
return date.ToDateTimeUtc().Ticks / TimeSpan.TicksPerMillisecond;
}
// 或者处理两个边界点
private static (long start, long end) DateRangeToTimestamps(BsonDate startDate, BsonDate endDate)
{
return (DateToTimestamp(startDate), DateToTimestamp(endDate));
}
```
4. 查询数据时,假设`YourDocument`是你文档中的模型类,包含`YourDateField`字段:
```csharp
var startTime = DateToTimestamp(new BsonDate(yourStartDateTime.ToUniversalTime()));
var endTime = DateToTimestamp(new BsonDate(yourEndDateTime.ToUniversalTime()));
var filter = Builders<YourDocument>.Filter.And(
Builders<YourDocument>.Filter.Gte("YourDateField", startTime),
Builders<YourDocument>.Filter.Lt("YourDateField", endTime));
var results = collection.Find(filter).ToList();
```
阅读全文
相关推荐
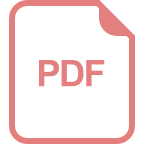
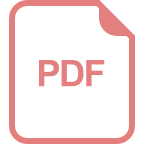
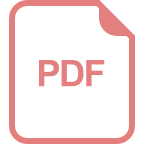
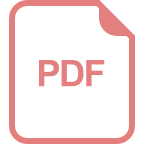
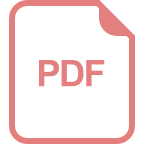
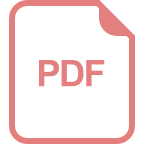
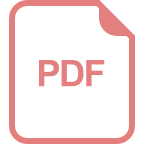
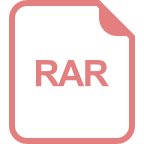
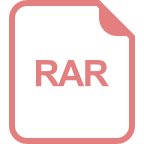
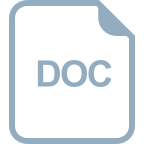
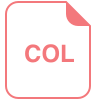
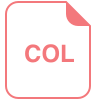
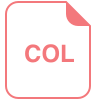
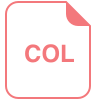
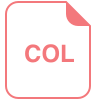

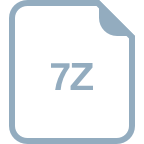