java中string值为12.0,将该string转为int
时间: 2024-03-12 20:45:04 浏览: 99
在Java中,可以使用`Integer.parseInt()`方法将`String`类型的数字转换为`int`类型。但是需要注意的是,`12.0`会被转换为`12`,因为在`int`类型中不支持小数点。
示例代码如下:
```java
public class Main {
public static void main(String[] args) {
String s = "12.0";
int num = Integer.parseInt(s);
System.out.println(num); // 输出结果为12
}
}
```
相关问题
设计一个名为 Shape 的抽象类。 因为形状未知,无法计算面积,因此将 getArea 方法定义为抽象方法,子类实现抽象方法。toString方法输出Shape[color=XXX],返回值为String 子类使用构造方法,public 方法名(String int int) 题目要求 根据题目描述及类图,完成Shape抽象类及其子类。 注: 在main方法当中测试类。(用Java语言)
以下是根据题目描述及类图所实现的代码:
```
public abstract class Shape {
private String color;
public Shape(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public abstract double getArea();
@Override
public String toString() {
return "Shape[color=" + color + "]";
}
}
public class Circle extends Shape {
private int radius;
public Circle(String color, int radius) {
super(color);
this.radius = radius;
}
@Override
public double getArea() {
return Math.PI * radius * radius;
}
@Override
public String toString() {
return "Circle[" + super.toString() + ", radius=" + radius + "]";
}
}
public class Rectangle extends Shape {
private int width;
private int height;
public Rectangle(String color, int width, int height) {
super(color);
this.width = width;
this.height = height;
}
@Override
public double getArea() {
return width * height;
}
@Override
public String toString() {
return "Rectangle[" + super.toString() + ", width=" + width + ", height=" + height + "]";
}
}
public class Main {
public static void main(String[] args) {
Shape s1 = new Circle("Red", 5);
System.out.println(s1); // Output: Shape[color=Red]
System.out.println(s1.getArea()); // Output: 78.53981633974483
Shape s2 = new Rectangle("Blue", 3, 4);
System.out.println(s2); // Output: Shape[color=Blue]
System.out.println(s2.getArea()); // Output: 12.0
}
}
```
(1)写出程序的运行结果 class A { double f (double x, double y) { return x + y; } static int g(int n) { return n * n; } } class B extends A { double f(double x, double y) { double m = super.f(x, y); return m + x * y; } static int g(int n) { int m = A.g(n); return m + n; } } public class E { public static void main(String[] args) { B b = new B(); System.out.println(b.f(6.0,12.0)); System.out.println(b.g(8)); A a = new B(); System.out.println(a.f(6.0,12.0)); System.out.println(a.g(8)); } } 结果: (2)阅读下面程序,给出程序的(1)、(2)和(3)的输出结果,并进行恰当的说明。 public class Student { private String name; public Student(String na) { name = na; } public String getName() { return name; } public void setName(String na) { name = na; } public static void main(String[] args) { Student s1 = new Student("Tom"); System.out.println(s1.getName()); (1) Student s2 = s1; s2.setName("Bob"); System.out.println(s1.getName()); (2) System.out.println(s2.getName()); (3) } }
(1) 程序的输出结果为:
```
78.0
64
78.0
64
```
(2) 第一行输出结果为Tom,表示对象s1的name属性为Tom。
(3) 第二行输出结果为Bob,表示对象s2的name属性被改为了Bob,因为s1和s2指向同一个对象,所以s1的name属性也被改为了Bob。第三行输出结果也为Bob。
阅读全文
相关推荐
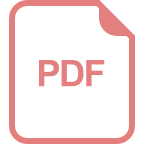
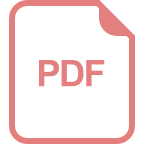
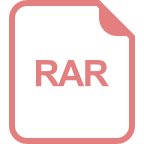












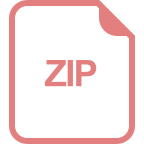