event instanceof ApplicationEvent启动后触发了5次的原因
时间: 2024-05-23 07:09:58 浏览: 9
`event instanceof ApplicationEvent`是一个判断`event`对象是否为`ApplicationEvent`实例的语句。在Spring Boot应用程序启动时,会触发多个`ApplicationEvent`事件,如`ApplicationStartingEvent`、`ApplicationEnvironmentPreparedEvent`、`ApplicationPreparedEvent`、`ApplicationStartedEvent`和`ApplicationReadyEvent`等。这些事件的数量可能会因为应用程序的配置和特性而不同。因此,如果`event instanceof ApplicationEvent`条件成立,就说明应用程序启动过程中触发了至少5个`ApplicationEvent`事件。
相关问题
event instanceof ApplicationEvent
这是一个Java代码片段,用于检查一个对象是否是ApplicationEvent的实例。
在Spring框架中,ApplicationEvent是一个用于表示应用程序中发生的事件的基本类。通常,我们使用ApplicationEvent的子类来定义自定义事件,并通过ApplicationEventPublisher将其发布给应用程序中的其他组件。
因此,使用"event instanceof ApplicationEvent"可以帮助我们检查一个对象是否是一个应用程序事件的实例。如果是,我们可以将其转换为一个具体的事件类型,并执行相关的操作。
java instanceof message instanceof EventMessage
在Java中,`instanceof`是一个用于检查对象是否属于特定类或其子类的运算符。它返回一个布尔值,如果对象是指定类的实例或其子类的实例,则返回`true`,否则返回`false`。
根据提供的引用内容,我们可以看到一个示例代码片段,其中使用了`instanceof`运算符来检查消息对象是否是`TextMessage`的实例。如果是,就将其转换为`TextMessage`类型,并打印出消息内容。
要回答你的问题,我们可以使用类似的方法来检查`message`对象是否是`EventMessage`的实例。下面是一个示例代码:
```java
if (message instanceof EventMessage) {
EventMessage eventMessage = (EventMessage) message;
// 处理EventMessage对象
System.out.println("message is an instance of EventMessage");
} else {
// 处理其他类型的消息对象
System.out.println("message is not an instance of EventMessage");
}
```
这段代码首先使用`instanceof`运算符检查`message`对象是否是`EventMessage`的实例。如果是,就将其转换为`EventMessage`类型,并执行相应的处理逻辑。如果不是,就执行其他类型的消息对象的处理逻辑。
相关推荐
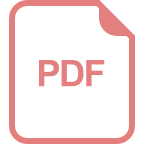
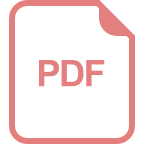











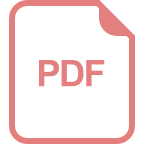