用python写一个用手机拍照并自动发送邮箱
时间: 2023-03-26 19:00:34 浏览: 155
您好,我可以回答这个问题。您可以使用Python中的OpenCV库来实现拍照功能,使用smtplib库来实现发送邮件功能。具体实现步骤可以参考以下代码:
```
import cv2
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
from email.mime.image import MIMEImage
# 拍照函数
def take_photo():
cap = cv2.VideoCapture()
ret, frame = cap.read()
cap.release()
return frame
# 发送邮件函数
def send_email(img_path):
# 邮件信息
sender = 'your_email_address'
receiver = 'recipient_email_address'
password = 'your_email_password'
subject = '自动发送的照片'
content = '这是一张自动发送的照片'
# 构造邮件
msg = MIMEMultipart()
msg['Subject'] = subject
msg['From'] = sender
msg['To'] = receiver
# 添加文本内容
text = MIMEText(content)
msg.attach(text)
# 添加图片附件
with open(img_path, 'rb') as f:
img_data = f.read()
img = MIMEImage(img_data)
img.add_header('Content-Disposition', 'attachment', filename='photo.jpg')
msg.attach(img)
# 发送邮件
try:
server = smtplib.SMTP_SSL('smtp.qq.com', 465)
server.login(sender, password)
server.sendmail(sender, receiver, msg.as_string())
server.quit()
print('邮件发送成功')
except Exception as e:
print('邮件发送失败')
print(e)
if __name__ == '__main__':
# 拍照
img = take_photo()
# 保存照片
cv2.imwrite('photo.jpg', img)
# 发送邮件
send_email('photo.jpg')
```
需要注意的是,发送邮件需要填写自己的邮箱地址、密码和收件人地址。另外,需要安装OpenCV和smtplib库。
阅读全文
相关推荐










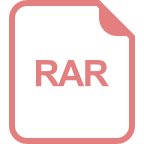







