python:主动关闭线程
时间: 2023-09-09 10:10:26 浏览: 92
可以使用threading模块中的Thread类的stop()方法来停止线程的执行,但这种方法并不推荐使用。另一种方法是使用一个标志来控制循环的执行,在需要停止线程时将标志设置为False。例如:
```python
import threading
class MyThread(threading.Thread):
def __init__(self):
super().__init__()
self._stop_event = threading.Event()
def stop(self):
self._stop_event.set()
def run(self):
while not self._stop_event.is_set():
# do something
pass
t = MyThread()
t.start()
# stop the thread after 10 seconds
t.join(10)
t.stop()
```
在这个示例中,线程的run()方法使用一个标志来控制循环的执行。当stop()方法被调用时,标志被设置为True,线程会在下一个循环中退出。在主线程中,使用join(timeout)方法来等待线程的结束,如果在timeout秒后线程仍未结束,就强制停止线程的执行。
相关问题
python主线程主动关闭子线程
如果你需要关闭子线程,你可以使用 threading 库的 stop() 方法,但是这个方法已经被弃用了。更好的方法是向子线程发送一个信号,让子线程自己停止。你可以使用一个标志变量,通过在子线程内不断检查该变量来实现这个目标。当主线程需要关闭子线程时,只需要将标志变量设置为 True 即可。子线程会在下一个循环中发现标志变量的状态已经变化,它会自己停止。这种方式是比较安全和可靠的方式来关闭子线程。
python 关闭线程
要关闭线程,可以使用 `threading.Thread` 的 `stop()` 方法。但是这种方法是不推荐使用的,因为它可能会导致线程在非预期的地方停止,从而引发不稳定的情况。
更好的方法是,让线程自己检查某个标志位,当检测到该标志位时,线程自己主动退出。具体实现可以参考以下代码:
```python
import threading
import time
class MyThread(threading.Thread):
def __init__(self):
super().__init__()
self._stop_event = threading.Event()
def stop(self):
self._stop_event.set()
def run(self):
while not self._stop_event.is_set():
print("Thread is running...")
time.sleep(1)
print("Thread stopped.")
# 创建线程并启动
t = MyThread()
t.start()
# 稍等一段时间后,手动停止线程
time.sleep(5)
t.stop()
```
在上述代码中,我们创建了一个名为 `MyThread` 的子类,其中包含一个 `_stop_event` 标志位。在 `run()` 方法中,线程会不断检查该标志位是否被设置,如果被设置,则主动退出循环,并打印出 "Thread stopped." 的提示信息。
在主程序中,我们创建了一个 `MyThread` 对象 `t`,并启动了该线程。然后等待 5 秒钟后,手动调用 `t.stop()` 方法,以停止线程的运行。
阅读全文
相关推荐
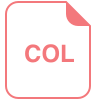
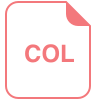
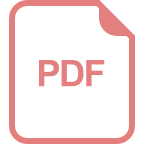
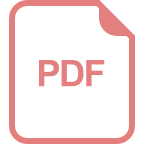
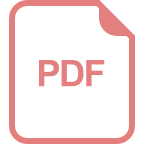
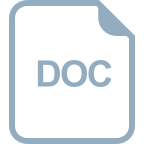
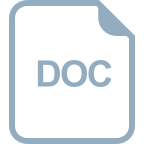
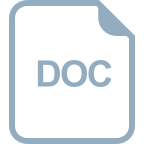
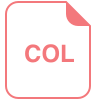
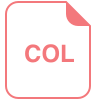
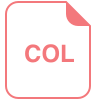
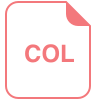
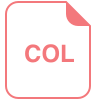