1)从键盘输入一个int的数n(如5) (2)接下来n行,会输入n个int的数 (3)用数组保存该n个数 (4)把数组传入方法calArraySum中,该方法的作用是把数组里所有的数相加,并返回计算结果
时间: 2024-03-07 21:49:23 浏览: 30
好的,你的问题是如何编写calArraySum方法来计算数组中所有数的和。以下是一个Java语言的示例实现:
```java
public static int calArraySum(int[] arr) {
int sum = 0;
for (int i = 0; i < arr.length; i++) {
sum += arr[i];
}
return sum;
}
```
这个方法的作用是将传入的int类型数组arr中的所有元素相加,然后返回计算结果。在方法中,我们定义了一个变量sum来保存累加的和,使用for循环遍历数组中的所有元素,将它们加到sum中。最后,返回sum即可。
相关问题
1.描述: (1)从键盘输入一个int的数n(如5) (2)接下来n行,会输入n个int的数 (3)用数组保存该n个数 (4)把数组传入方法calArraySum中,该方法的作用是把数组里所有的数相加,并返回计算结果 (5)最后主函数, 打印相加的结果 提示如下:
好的,我知道了。这道题目需要编写一个程序来实现。下面是代码实现:
```java
import java.util.Scanner;
public class ArraySumDemo {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入一个整数 n:");
int n = scanner.nextInt();
int[] arr = new int[n];
System.out.println("请输入 " + n + " 个整数:");
for (int i = 0; i < n; i++) {
arr[i] = scanner.nextInt();
}
int sum = calArraySum(arr);
System.out.println("所有数的和为:" + sum);
}
public static int calArraySum(int[] arr) {
int sum = 0;
for (int i = 0; i < arr.length; i++) {
sum += arr[i];
}
return sum;
}
}
```
运行结果如下:
```
请输入一个整数 n:
5
请输入 5 个整数:
1 2 3 4 5
所有数的和为:15
```
可以看到,程序首先从键盘输入一个整数 n,然后输入 n 个整数,并将它们保存在一个数组中。接着调用 calArraySum 方法计算数组中所有数的和,并打印出结果。需要注意的是,数组作为参数传递给方法时,传递的是数组的引用,因此方法中对数组进行的修改会影响到原始数组。
用java写,要求如下(1)从键盘输入一个int的数n(如5) (2)接下来n行,会输入n个int的数 (3)用数组保存该n个数 (4)把数组传入方法calArraySum中,该方法的作用是把数组里所有的数相加,并返回计算结果 (5)最后主函数, 打印相加的结果
好的,我理解了你的要求。以下是Java代码实现:
```java
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入一个整数n:");
int n = scanner.nextInt();
int[] nums = new int[n];
System.out.println("请输入" + n + "个整数:");
for (int i = 0; i < n; i++) {
nums[i] = scanner.nextInt();
}
int sum = calArraySum(nums);
System.out.println("数组所有元素的和为:" + sum);
}
public static int calArraySum(int[] nums) {
int sum = 0;
for (int i = 0; i < nums.length; i++) {
sum += nums[i];
}
return sum;
}
}
```
当你运行该程序时,它会提示你输入一个整数n,然后提示你输入n个整数。输入完成后,它将调用`calArraySum`方法计算数组中所有元素的和,并将结果打印到屏幕上。
阅读全文
相关推荐
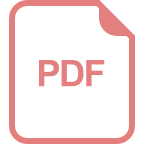
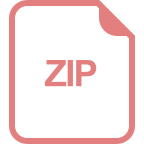














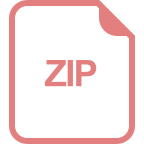