#!/usr/local/bin/python3 # -*- coding: utf-8 -*- # @Time : 2023/6/11 14:19 # @Author : 志在星空 # @File : jietu12.py # @Software: PyCharm import base64 import urllib import requests import json API_KEY = "jHxdMDf2bhEPxcG6zFoRUejo" SECRET_KEY = "QF5GO9eLQncG2Zr1MKrxLOWvKAkAtVfI" def main(): # 发送 OCR 请求并获取响应 url = "https://aip.baidubce.com/rest/2.0/ocr/v1/general?access_token=" + get_access_token() # payload = 'image=%2F9j%2F4AAQ' headers = { 'Content-Type': 'application/x-www-form-urlencoded', 'Accept': 'application/json' } image_path = "11.jpg" with open(image_path, "rb") as image_file: image_data = image_file.read() response = requests.post(url, headers=headers, data=image_data) # response = requests.request("POST", url, headers=headers, data=payload) # 解析响应并提取 words_result 中的所有 words 字段 result = json.loads(response.text) print(result) # words_list = [item['words'] for item in result['words_result']] # print(words_list) # # # 打印所有提取到的内容 # for words in words_list: # print(words) def get_file_content_as_base64(path, urlencoded=False): """ 获取文件base64编码 :param path: 文件路径 :param urlencoded: 是否对结果进行urlencoded :return: base64编码信息 """ with open(path, "rb") as f: content = base64.b64encode(f.read()).decode("utf8") if urlencoded: content = urllib.parse.quote_plus(content) return content def get_access_token(): """ 使用 AK,SK 生成鉴权签名(Access Token) :return: access_token,或是None(如果错误) """ url = "https://aip.baidubce.com/oauth/2.0/token" params = {"grant_type": "client_credentials", "client_id": API_KEY, "client_secret": SECRET_KEY} return str(requests.post(url, params=params).json().get("access_token")) if __name__ == '__main__': main()运行出现{'log_id': 1667825949995168966, 'error_msg': 'param image not exist', 'error_code': 216101},怎么办
时间: 2024-03-30 17:40:33 浏览: 17
根据API的响应信息,错误信息为"param image not exist",也就是说API无法找到你指定的图片文件。请确保文件路径是正确的,并且文件名和扩展名的大小写与实际文件匹配。如果文件名或路径错误,将导致API无法找到该文件并出现上述错误。如果你确定文件路径和文件名正确无误,那么你可以检查一下API文档以确定API对请求的其他要求,例如请求头、请求参数等是否正确。此外,你还可以尝试使用图片的base64编码代替图片路径传递给API,方法详见上面代码中的`get_file_content_as_base64`函数。
相关问题
#!/usr/bin/tcsh -f 与#!/usr/bin/tcsh的区别
#!/usr/bin/tcsh -f 与#!/usr/bin/tcsh的区别在于是否使用了"-f"参数。
#!/usr/bin/tcsh -f:使用了"-f"参数,表示在执行脚本时,不会读取用户的配置文件(例如~/.tcshrc),从而跳过配置文件中的设置,直接执行脚本中的命令。
#!/usr/bin/tcsh:没有使用"-f"参数,表示在执行脚本时,会读取用户的配置文件,并按照配置文件中的设置执行脚本中的命令。
使用"-f"参数可以确保脚本的执行与环境设置无关,对于一些需要独立环境的脚本来说,这样的设置可能更加可靠。然而,有些情况下,可能需要加载用户的配置文件来获取特定的环境变量或别名设置等。
所以,要根据具体的需求来决定是否使用"-f"参数。
#!/usr/bin/expect -f和#!/bin/bash
引用中给出了一个脚本的示例,其中#!/usr/bin/expect -f是脚本的第一行,用来指定使用expect作为脚本的解释器。这意味着这个脚本将使用expect的语法和功能来执行。而#!/bin/bash是另一种脚本的解释器,用于指定使用bash作为脚本的解释器。bash是一种常用的Unix shell程序,用于执行命令和脚本。
简而言之,#!/usr/bin/expect -f用于指定使用expect解释器来执行脚本,而#!/bin/bash用于指定使用bash解释器来执行脚本。两者都是根据脚本的需要来选择合适的解释器。<em>1</em><em>2</em><em>3</em>
#### 引用[.reference_title]
- *1* *2* *3* [shell阶段-day12-expect](https://blog.csdn.net/qq_23995091/article/details/113571554)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT3_1"}} ] [.reference_item]
[ .reference_list ]
相关推荐
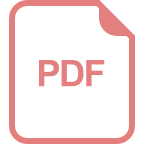
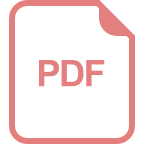
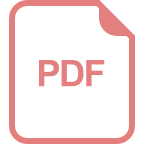













