用c++,输入5个学生的信息(包括学号、姓名、成绩),要求按照成绩的高低顺序输出各学生的信息。要求用结构体数组定义学生的信息。
时间: 2024-03-16 18:46:14 浏览: 68
好的,我可以为您解答这个问题。
以下是示例代码:
```c++
#include <iostream>
#include <algorithm> //用于排序
using namespace std;
struct Student {
int id;
string name;
float score;
};
bool cmp(Student a, Student b) { //比较函数
return a.score > b.score;
}
int main() {
Student stu[5]; //定义结构体数组
for(int i = 0; i < 5; i++) {
cout << "请输入第" << i+1 << "个学生的信息:\n";
cout << "学号:";
cin >> stu[i].id;
cout << "姓名:";
cin >> stu[i].name;
cout << "成绩:";
cin >> stu[i].score;
}
sort(stu, stu+5, cmp); //按成绩从高到低排序
cout << "按成绩从高到低输出学生信息:\n";
for(int i = 0; i < 5; i++) {
cout << "学号:" << stu[i].id << "\t姓名:" << stu[i].name << "\t成绩:" << stu[i].score << endl;
}
return 0;
}
```
这个程序首先定义了一个 `Student` 结构体,包含三个成员变量:`id`、`name`、`score`,分别表示学号、姓名和成绩。然后通过结构体数组定义了五个学生的信息。接着利用 `sort` 函数将学生按照成绩从高到低排序,排序时需要传入一个比较函数 `cmp`,该函数的作用是比较两个学生的成绩大小。最后按照排序后的顺序输出学生信息。
希望这个示例代码能够帮助您解决问题。
相关推荐
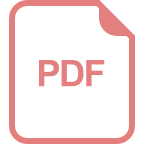
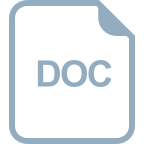
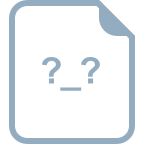














