13:将字符串中的小写字母转换成大写字母c++
时间: 2024-05-04 17:22:15 浏览: 113
可以使用标准库函数 `toupper()` 将字符串中的小写字母转换成大写字母。下面是一个使用 `toupper()` 函数的示例代码:
```c++
#include <iostream>
#include <string>
#include <cctype>
int main() {
std::string str = "Hello, World!";
for (auto& c : str) {
if (std::islower(c)) {
c = std::toupper(c);
}
}
std::cout << str << std::endl;
return 0;
}
```
输出结果为:
```
HELLO, WORLD!
```
这里使用了 `std::islower()` 判断字符是否为小写字母,如果是,则使用 `std::toupper()` 函数将其转换成大写字母。循环遍历字符串中的所有字符,将所有的小写字母都转换成大写字母。
相关问题
C++中字符串中小写字母转换为大写字母
在C++中,你可以使用标准库提供的`std::toupper`函数将字符串中的小写字母转换为大写字母。这个函数需要一个字符作为输入,如果它是小写字母,它会返回对应的大写字母;如果不是,就保持原样。不过需要注意的是,`std::toupper`是针对单个字符操作的。
如果你有一个字符串想批量转换,可以遍历字符串中的每个字符,然后应用`std::toupper`。例如:
```cpp
#include <cctype> // 包含 toupper 函数
#include <string>
std::string toUpper(const std::string &str) {
std::string result = str;
for (char& c : result) {
if (islower(c)) { // 检查字符是否为小写
c = toupper(c); // 转换为大写
}
}
return result;
}
```
如果你想直接修改原始字符串而不是创建新副本,可以使用`std::transform`和范围for循环:
```cpp
#include <algorithm>
#include <cctype>
void toUpperInPlace(std::string &str) {
std::transform(str.begin(), str.end(), str.begin(), ::toupper);
}
```
将字符串中的小写字母转换成大写字母c++
可以使用 C++ 中的标准库函数 `toupper`,它可以将小写字母转换成大写字母。具体实现如下:
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
string str = "Hello, World!";
for (int i = 0; i < str.size(); i++) {
if (islower(str[i])) {
str[i] = toupper(str[i]);
}
}
cout << str << endl; // 输出 HELLO, WORLD!
return 0;
}
```
在上面的代码中,我们先判断字符串中每个字符是否为小写字母,如果是,则使用 `toupper` 将其转换为大写字母。最后输出转换后的字符串。
阅读全文
相关推荐
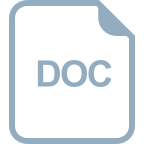
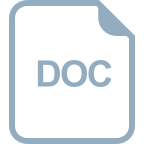
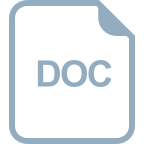
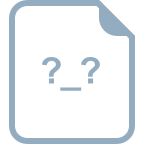
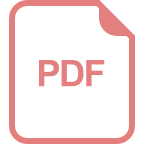











