uniapp上拉加载更多防抖节流
时间: 2023-09-02 12:12:20 浏览: 135
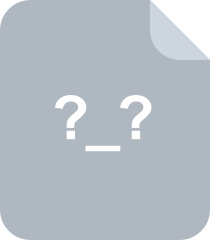
uniapp实现上拉刷新下拉加载
在uniapp中,可以使用debounce和throttle两种方式来实现上拉加载更多的防抖和节流。
1. 防抖
防抖是指在一段时间内只执行一次函数,常用于防止频繁触发函数。在上拉加载更多的场景中,可以使用防抖来避免用户快速滑动页面时频繁加载数据。
示例代码:
```javascript
// 防抖函数
function debounce(fn, delay) {
let timer = null;
return function() {
clearTimeout(timer);
timer = setTimeout(() => {
fn.apply(this, arguments);
}, delay);
}
}
// 上拉加载更多
export default {
data() {
return {
list: [],
page: 1,
limit: 10,
isLoading: false
}
},
methods: {
// 加载数据
loadData() {
// 避免重复加载
if (this.isLoading) return;
this.isLoading = true;
// 请求数据
api.getList(this.page, this.limit).then(res => {
this.list = this.list.concat(res.data);
this.page++;
this.isLoading = false;
});
},
// 监听滚动事件
onScroll() {
const scrollTop = uni.getSystemInfoSync().scrollTop;
const windowHeight = uni.getSystemInfoSync().windowHeight;
const scrollHeight = uni.getSystemInfoSync().scrollHeight;
// 判断是否滑到底部
if (scrollTop + windowHeight >= scrollHeight) {
this.loadData = debounce(this.loadData, 500);
this.loadData();
}
}
}
}
```
在上面的代码中,我们使用了debounce函数来包装loadData函数,设置了500ms的延迟时间,避免用户快速滑动页面时频繁加载数据。
2. 节流
节流是指在一段时间内只执行一次函数,常用于优化频繁触发函数的性能。在上拉加载更多的场景中,可以使用节流来控制数据的加载频率,避免服务器压力过大。
示例代码:
```javascript
// 节流函数
function throttle(fn, delay) {
let timer = null;
return function() {
if (!timer) {
timer = setTimeout(() => {
fn.apply(this, arguments);
timer = null;
}, delay);
}
}
}
// 上拉加载更多
export default {
data() {
return {
list: [],
page: 1,
limit: 10,
isLoading: false
}
},
methods: {
// 加载数据
loadData() {
// 避免重复加载
if (this.isLoading) return;
this.isLoading = true;
// 请求数据
api.getList(this.page, this.limit).then(res => {
this.list = this.list.concat(res.data);
this.page++;
this.isLoading = false;
});
},
// 监听滚动事件
onScroll() {
const scrollTop = uni.getSystemInfoSync().scrollTop;
const windowHeight = uni.getSystemInfoSync().windowHeight;
const scrollHeight = uni.getSystemInfoSync().scrollHeight;
// 判断是否滑到底部
if (scrollTop + windowHeight >= scrollHeight) {
this.loadData = throttle(this.loadData, 500);
this.loadData();
}
}
}
}
```
在上面的代码中,我们使用了throttle函数来包装loadData函数,设置了500ms的间隔时间,确保每500ms只加载一次数据,避免服务器压力过大。
阅读全文
相关推荐
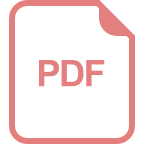
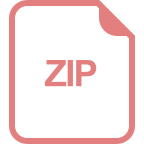







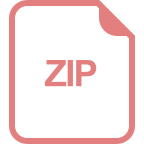
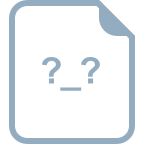
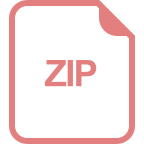


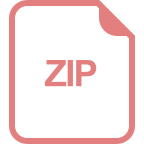