切面获取方法参数
时间: 2023-08-18 14:13:33 浏览: 116
在 Spring AOP 中,可以使用 `JoinPoint` 参数来获取连接点(方法调用)信息,包括方法名、参数等。具体可以通过以下方式获取方法参数:
```java
@Aspect
@Component
public class MyAspect {
@Before("execution(* com.example.demo.controller.TestController.test(..))")
public void before(JoinPoint joinPoint) {
Object[] args = joinPoint.getArgs();
for(Object arg : args) {
// 处理参数
}
}
}
```
在上述代码中,我们使用了 `JoinPoint` 参数来获取连接点信息,并使用 `joinPoint.getArgs()` 方法来获取方法参数,返回值为 `Object[]` 数组类型,其中每个元素表示一个方法参数。可以根据实际需要进行处理。
相关问题
切面获取方法中的参数
在切面编程(AOP, Aspect-Oriented Programming)中,当你需要在某个特定方法的执行前后或者仅当满足某些条件时执行额外的操作,比如日志记录、权限验证等,这时就需要通过切面去拦截并获取方法中的参数。在Spring AOP中,可以使用`@Around`注解的 advisors 来实现这一点。
`@Around`的处理程序(advice)是一个环绕通知(around advice),它会在目标方法执行前和执行后分别执行。你可以使用`proceed()`方法来调用目标方法,并通过`JoinPoint`对象的`getArgs()`方法来访问传入的方法参数。例如:
```java
@Aspect
@Component
public class LoggingAspect {
@Around("execution(* com.example.service.*.*(..))")
public Object logAndAuthorize(ProceedingJoinPoint joinPoint) throws Throwable {
// 获取方法参数
Object[] args = joinPoint.getArgs();
System.out.println("Before method execution with arguments: " + Arrays.toString(args));
// 执行原方法
Object result = joinPoint.proceed();
// 其他操作...
return result;
}
}
```
在这个例子中,`execution(* com.example.service.*.*(..))`是一个切入点表达式,匹配所有com.example.service包下的类的方法。`args`变量就是传递给方法的所有参数。你需要根据实际需求来访问和处理这些参数。
aop切面获取请求参数
```java
// AOP切面获取请求参数示例
@Aspect
@Component
public class RequestParamAspect {
@Around("@annotation(com.example.RequestLog)")
public Object logRequest(ProceedingJoinPoint joinPoint) throws Throwable {
// 获取方法参数
Object[] args = joinPoint.getArgs();
// 记录请求参数
for (Object arg : args) {
// 处理参数记录逻辑
System.out.println("Request parameter: " + arg.toString());
}
// 执行原方法
Object result = joinPoint.proceed();
return result;
}
}
```
阅读全文
相关推荐
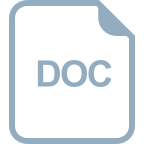
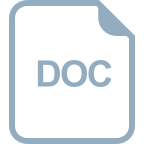
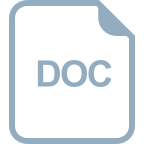













