用Java多线程,集合,网络通信写一个租车App
时间: 2023-07-01 14:04:28 浏览: 129
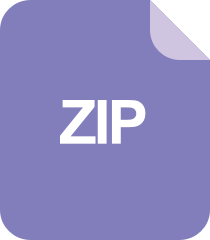
Java期末大作业 基于Java+JSP+Servlet实现的租车管理系统源码

好的,下面是一个简单的租车App的Java代码示例:
```java
import java.io.IOException;
import java.net.ServerSocket;
import java.net.Socket;
import java.util.ArrayList;
import java.util.List;
public class RentCarAppServer {
private static final int PORT = 8888;
private static List<ClientHandler> clients = new ArrayList<>();
public static void main(String[] args) throws IOException {
ServerSocket serverSocket = new ServerSocket(PORT);
System.out.println("Server started...");
while(true) {
Socket clientSocket = serverSocket.accept();
System.out.println("Client connected: " + clientSocket);
ClientHandler clientHandler = new ClientHandler(clientSocket, clients);
clients.add(clientHandler);
clientHandler.start();
}
}
}
class ClientHandler extends Thread {
private Socket clientSocket;
private List<ClientHandler> clients;
private String name;
private boolean isRented = false;
public ClientHandler(Socket clientSocket, List<ClientHandler> clients) {
this.clientSocket = clientSocket;
this.clients = clients;
}
@Override
public void run() {
try {
handleClient();
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
clientSocket.close();
} catch (IOException e) {
e.printStackTrace();
}
clients.remove(this);
System.out.println("Client disconnected: " + clientSocket);
}
}
private void handleClient() throws IOException {
String inputLine;
while ((inputLine = CommunicationUtils.receiveMessage(clientSocket)) != null) {
if (name == null) {
name = inputLine;
CommunicationUtils.sendMessage(clientSocket, "Welcome " + name + "!");
broadcastMessage(name + " has joined the chat!");
} else {
if (inputLine.startsWith("rent")) {
if (isRented) {
CommunicationUtils.sendMessage(clientSocket, "You have already rented a car.");
} else {
isRented = true;
broadcastMessage(name + " has rented a car.");
}
} else if (inputLine.startsWith("return")) {
if (!isRented) {
CommunicationUtils.sendMessage(clientSocket, "You have not rented any car yet.");
} else {
isRented = false;
broadcastMessage(name + " has returned a car.");
}
} else {
broadcastMessage(name + ": " + inputLine);
}
}
}
}
private void broadcastMessage(String message) {
for (ClientHandler client : clients) {
if (client != this) {
CommunicationUtils.sendMessage(client.clientSocket, message);
}
}
}
}
class CommunicationUtils {
public static String receiveMessage(Socket socket) throws IOException {
return new String(socket.getInputStream().readAllBytes()).trim();
}
public static void sendMessage(Socket socket, String message) throws IOException {
socket.getOutputStream().write((message + "\n").getBytes());
}
}
```
这个租车App使用了Java的多线程机制,每当有一个客户端连接时,就会启动一个新的线程处理该客户端的请求。同时,该App使用了Java集合来存储所有客户端的信息,并使用了Java网络通信来实现客户端与服务器之间的通信。
客户端可以发送 "rent" 和 "return" 命令来租借和归还车辆,同时还可以发送普通文本消息来与其他客户端进行聊天。
需要注意的是,这只是一个简单的示例代码,实际的租车App需要更加完善的功能和更严谨的代码。
阅读全文
相关推荐
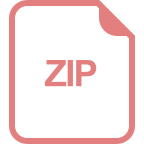

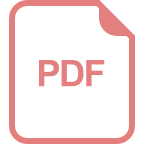
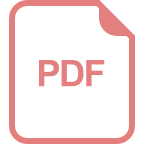
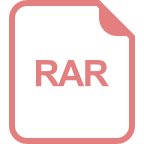
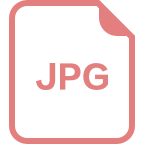
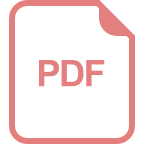
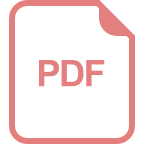
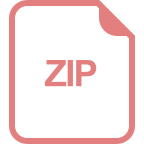
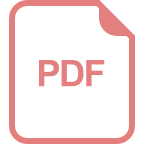
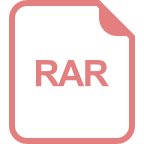
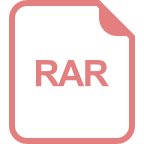
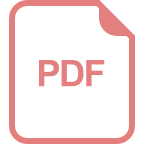
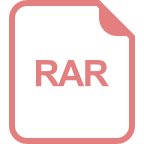
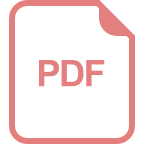