将字符串大写转为小写,小写转换为大写
时间: 2023-09-29 07:02:45 浏览: 47
可以使用Python内置函数 `upper()` 将小写字符串转换为大写字符串,使用 `lower()` 将大写字符串转换为小写字符串。下面是一个示例代码:
```python
str = "Hello World"
print(str.upper()) # 输出 "HELLO WORLD"
print(str.lower()) # 输出 "hello world"
```
你可以根据自己的需求将上面的示例代码进行修改。
相关问题
c语言怎么将字符串中的小写转换为大写
在C语言中,可以使用标准库函数 `toupper()` 将字符串中的小写字母转换为大写字母。具体操作步骤如下:
1. 引入头文件 `ctype.h`。
2. 使用 `toupper()` 函数将字符串中的字符逐一转换为大写字母。
下面是一个简单的示例代码:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char str[] = "hello world";
int i = 0;
while (str[i]) {
str[i] = toupper(str[i]);
i++;
}
printf("%s", str);
return 0;
}
```
输出结果为:
```
HELLO WORLD
```
在上述代码中,使用了 `toupper()` 函数将字符串中的小写字母转换为大写字母,并将转换后的字符串输出。
c语言将字符串小写转换为大写
可以使用C语言中的toupper函数将小写字母转换为大写字母。下面是一个简单的示例代码:
```
#include <stdio.h>
#include <ctype.h>
int main() {
char str[] = "hello world";
int i;
printf("Original string: %s\n", str);
for (i = 0; str[i]; i++) {
str[i] = toupper(str[i]);
}
printf("Uppercase string: %s\n", str);
return 0;
}
```
在这个示例中,我们首先定义了一个字符串`str`,然后使用`toupper`函数将其中的小写字母转换为大写字母,并将结果存储回原始字符串中。最后,我们打印出转换后的字符串。
相关推荐
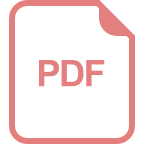
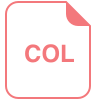










