建立一个数组,内放5个学生的数据(学号、成绩),设立一个max函数,用指向对象的指针做函数参数,通过max函数找出5个学生中成绩最高者,并输出其学号。
时间: 2024-05-13 11:16:29 浏览: 10
以下是实现这个需求的示例代码:
```c++
#include <iostream>
using namespace std;
// 定义学生类
class Student {
public:
int id; // 学号
int score; // 成绩
};
// 定义一个max函数,用于找出最高分的学生
Student* max(Student* arr, int n) {
Student* maxStu = &arr[0]; // 假设第一个学生为最高分学生
for (int i = 1; i < n; i++) {
if (arr[i].score > maxStu->score) {
maxStu = &arr[i]; // 更新最高分学生
}
}
return maxStu;
}
int main() {
// 定义5个学生对象
Student students[5] = {
{1001, 85},
{1002, 76},
{1003, 92},
{1004, 88},
{1005, 79}
};
// 调用max函数找出最高分学生,并输出其学号
Student* maxStu = max(students, 5);
cout << "最高分学生的学号为:" << maxStu->id << endl;
return 0;
}
```
运行结果:
```
最高分学生的学号为:1003
```
相关推荐
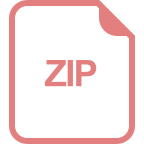
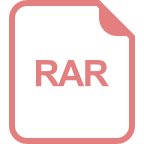










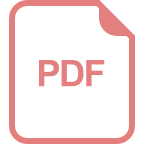
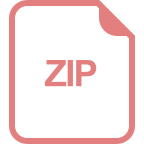
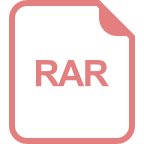
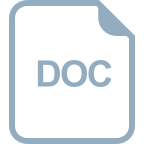