遍历set容器
时间: 2023-07-03 16:14:13 浏览: 110
遍历set容器可以使用迭代器,代码示例如下:
```cpp
#include <iostream>
#include <set>
int main() {
std::set<int> myset = {1, 2, 3, 4, 5};
for (auto it = myset.begin(); it != myset.end(); ++it) {
std::cout << *it << " ";
}
std::cout << std::endl;
return 0;
}
```
以上代码会输出 `1 2 3 4 5`,即set容器中的所有元素。
相关问题
遍历c++set容器和map容器
在C++中,可以使用迭代器来遍历set容器和map容器。下面是遍历这两种容器的示例代码:
遍历set容器:
```cpp
std::set<int> mySet = {1, 2, 3, 4, 5};
// 使用迭代器遍历set容器
for (std::set<int>::iterator it = mySet.begin(); it != mySet.end(); ++it) {
int element = *it; // 获取当前元素的值
// 执行操作,例如打印或处理元素
}
```
遍历map容器:
```cpp
std::map<int, std::string> myMap = {{1, "apple"}, {2, "banana"}, {3, "cherry"}};
// 使用迭代器遍历map容器
for (std::map<int, std::string>::iterator it = myMap.begin(); it != myMap.end(); ++it) {
int key = it->first; // 获取当前键的值
std::string value = it->second; // 获取当前值的值
// 执行操作,例如打印或处理键值对
}
```
另外,C++11引入了范围-based for循环,也可以用于遍历set容器和map容器:
遍历set容器:
```cpp
std::set<int> mySet = {1, 2, 3, 4, 5};
// 使用范围-based for循环遍历set容器
for (const auto& element : mySet) {
// 执行操作,例如打印或处理元素
}
```
遍历map容器:
```cpp
std::map<int, std::string> myMap = {{1, "apple"}, {2, "banana"}, {3, "cherry"}};
// 使用范围-based for循环遍历map容器
for (const auto& pair : myMap) {
int key = pair.first; // 获取当前键的值
std::string value = pair.second; // 获取当前值的值
// 执行操作,例如打印或处理键值对
}
```
请注意,在遍历map容器时,使用`pair.first`获取键,使用`pair.second`获取值。
迭代器遍历map容器
在JavaScript中,可以使用迭代器来遍历Map容器中的元素。迭代器是一个实现了@@iterator方法的对象,它通过调用next()方法来依次返回Map中的键值对。
以下是一个示例代码,展示了如何使用迭代器遍历Map容器:
```javascript
// 创建一个Map容器
const map = new Map();
map.set("key1", "value1");
map.set("key2", "value2");
map.set("key3", "value3");
// 获取Map的迭代器对象
const iterator = map();
// 使用迭代器遍历Map容器
let result = iterator.next();
while (!result.done) {
console.log(result.value); // 输出键值对
result = iterator.next(); // 获取下一个键值对
}
```
在上述代码中,通过调用Map容器的[Symbol.iterator]()方法,可以获取到一个迭代器对象。然后使用while循环和next()方法遍历Map容器,直到迭代器的done属性为true时停止循环。每次循环中,可以通过迭代器的value属性获取到当前的键值对。
希望这个例子能够帮助你理解如何使用迭代器遍历Map容器。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
阅读全文
相关推荐
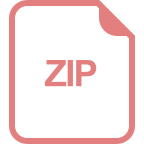
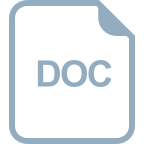











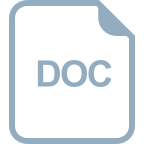

