linux select判断fd_set个数
时间: 2024-01-14 14:03:57 浏览: 33
在使用 `select` 函数进行 I/O 多路复用时,需要对返回值进行判断以确定有哪些文件描述符就绪。`select` 函数的返回值表示就绪文件描述符的数量,如果返回值为 0,则说明在超时时间内没有文件描述符就绪;如果返回值为 -1,则说明 `select` 函数调用失败。
在判断就绪的文件描述符时,可以使用 `FD_ISSET` 函数对每个文件描述符进行判断。`FD_ISSET` 函数返回值为非零表示该文件描述符存在于集合中,为零表示不存在于集合中。
如果需要统计有多少个文件描述符就绪,可以遍历文件描述符集合,对每个文件描述符调用 `FD_ISSET` 函数进行判断,并累加计数。
下面是一个简单的示例代码,用于统计有多少个文件描述符就绪:
```c
#include <stdio.h>
#include <stdlib.h>
#include <sys/select.h>
#include <unistd.h>
int main() {
fd_set rfds; // 用于存储文件描述符集合的 fd_set 结构体
int maxfd = STDIN_FILENO + 1; // 待监视的最大文件描述符值加一
struct timeval timeout = {5, 0}; // 超时时间为 5 秒
while (1) {
FD_ZERO(&rfds); // 将读文件描述符集合清零
FD_SET(STDIN_FILENO, &rfds); // 将标准输入加入到读文件描述符集合
// 调用 select 函数进行 I/O 多路复用
int ret = select(maxfd, &rfds, NULL, NULL, &timeout);
if (ret < 0) {
perror("select error");
exit(1);
} else if (ret == 0) {
printf("timeout\n");
} else {
int count = 0;
// 遍历文件描述符集合,统计有多少个文件描述符就绪
for (int fd = 0; fd < maxfd; fd++) {
if (FD_ISSET(fd, &rfds)) {
count++;
}
}
printf("%d file descriptor(s) are ready\n", count);
// 如果标准输入可读,进行读操作
if (FD_ISSET(STDIN_FILENO, &rfds)) {
char buf[1024];
int n = read(STDIN_FILENO, buf, sizeof(buf));
if (n < 0) {
perror("read error");
exit(1);
} else if (n == 0) {
printf("EOF\n");
break;
} else {
printf("read: %s", buf);
}
}
}
}
return 0;
}
```
在该程序中,当 `select` 函数返回时,程序首先遍历文件描述符集合,统计有多少个文件描述符就绪,然后通过 `FD_ISSET` 函数判断标准输入是否可读,如果可读则进行读操作。
相关推荐
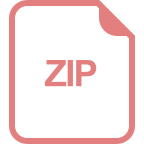
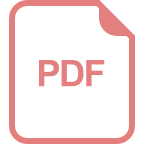
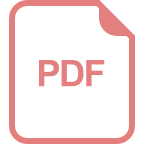














