java中判断控制台中输入的单词是否与在文件中出现,并写上详细的注释
时间: 2023-06-03 18:06:27 浏览: 58
好的,这是一个技术问题,可以回答。以下是判断控制台中输入的单词是否与在文件中出现并附带详细注释的Java代码:
```java
import java.io.File;
import java.io.IOException;
import java.util.Scanner;
public class WordInFileChecker {
public static void main(String[] args) {
Scanner console = new Scanner(System.in);
System.out.print("Enter a word to search for in the file: ");
String searchWord = console.nextLine();
File file = new File("sample.txt");
try {
Scanner fileScanner = new Scanner(file);
boolean found = false;
while (fileScanner.hasNextLine()) {
String line = fileScanner.nextLine();
if (line.contains(searchWord)) { // 判断行是否包含搜索单词
found = true;
break;
}
}
if (found) {
System.out.println("The word " + searchWord + " was found in the file.");
} else {
System.out.println("The word " + searchWord + " was not found in the file.");
}
fileScanner.close();
} catch (IOException e) {
System.out.println("An error occurred: " + e.getMessage());
}
}
}
```
注释:
- `import java.io.File;`:导入 Java 的文件处理类库。
- `import java.io.IOException;`:导入 Java 的输入/输出异常处理类库。
- `import java.util.Scanner;`:导入 Java 的输入类库。
- `Scanner console = new Scanner(System.in);`:创建一个新的 Scanner 对象,以便从控制台中读取输入。
- `System.out.print("Enter a word to search for in the file: ");`:提示用户输入要搜索的单词。
- `String searchWord = console.nextLine();`:读取用户从控制台输入的单词。
- `File file = new File("sample.txt");`:创建一个指向名为 "sample.txt" 的文件的文件对象。
- `Scanner fileScanner = new Scanner(file);`:创建一个新的 Scanner 对象,以便从文件中读取输入。
- `while (fileScanner.hasNextLine()) {`:遍历文件中的每一行。
- `String line = fileScanner.nextLine();`:读取文件中的下一行。
- `if (line.contains(searchWord)) {`:检查当前行是否包含搜索单词。
- `found = true;`:如果搜索单词在当前行中出现,则将 found 标记为 true。
- `break;`:如果找到了搜索单词,则退出循环。
- `if (found) {`:如果搜索单词在文件中出现过,则执行以下代码。
- `System.out.println("The word " + searchWord + " was found in the file.");`:打印包含搜索单词的文件中的第一行。
- `else { System.out.println("The word " + searchWord + " was not found in the file.");`:否则,表示搜索单词未在文件中找到。
- `fileScanner.close();`:关闭文件扫描器。
- `catch (IOException e) { System.out.println("An error occurred: " + e.getMessage()); }`:如果读取文件时发生异常,则打印错误消息。
相关推荐
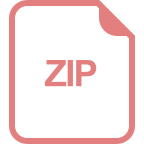














