tf.variable_scope用法
时间: 2023-05-17 10:04:26 浏览: 195
tf.variable_scope用于创建变量作用域,可以在同一个作用域内共享变量。使用tf.get_variable()函数创建变量时,可以指定变量所在的作用域。例如:
```
with tf.variable_scope('my_scope'):
var1 = tf.get_variable('var1', shape=[2, 3])
var2 = tf.get_variable('var2', shape=[3, 4])
```
在这个例子中,var1和var2都在名为'my_scope'的作用域内。如果在同一个作用域内再次定义同名的变量,会抛出异常。
需要注意的是,如果在作用域内使用tf.get_variable()创建变量,那么变量名必须是唯一的,否则会抛出异常。如果想要共享变量,可以使用tf.variable_scope()的reuse参数,例如:
```
with tf.variable_scope('my_scope'):
var1 = tf.get_variable('var1', shape=[2, 3])
tf.get_variable_scope().reuse_variables()
var2 = tf.get_variable('var1')
```
在这个例子中,var1和var2共享同一个变量。
相关问题
tf.keras.layers.Layer怎么替代variable_scope
在TensorFlow 2.0中,`tf.keras.layers.Layer`已经默认使用了变量共享,无需手动使用`variable_scope`。
在TensorFlow 1.x中,可以通过以下方式实现`variable_scope`的功能:
1. 在自定义层的`__init__`方法中,使用`tf.variable_scope`创建域,并将其赋值给`self.scope`:
```python
class MyLayer(tf.keras.layers.Layer):
def __init__(self, name, **kwargs):
super(MyLayer, self).__init__(name=name, **kwargs)
self.scope = tf.variable_scope(name)
```
2. 在自定义层的`call`方法中,使用`tf.variable_scope`进入该域,并使用`tf.get_variable`创建或获取变量:
```python
class MyLayer(tf.keras.layers.Layer):
def __init__(self, name, **kwargs):
super(MyLayer, self).__init__(name=name, **kwargs)
self.scope = tf.variable_scope(name)
def call(self, inputs):
with self.scope:
weight = tf.get_variable(name='weight', shape=[inputs.shape[-1], 10], initializer=tf.random_normal_initializer())
bias = tf.get_variable(name='bias', shape=[10], initializer=tf.zeros_initializer())
output = tf.matmul(inputs, weight) + bias
return output
```
这样就实现了类似于`variable_scope`的功能。但是在TensorFlow 2.0中,不再需要手动创建域,可以直接使用`tf.get_variable`创建或获取变量。
if training: # each agent has their own var_scope var_scope = ('actor_agent_%02d'%self.n_agent)
这段代码是针对多智能体强化学习场景中的一个智能体,为其定义了一个 TensorFlow 中的变量作用域(variable scope),并将其命名为 `actor_agent_xx`,其中 `xx` 表示智能体编号。在多智能体场景中,每个智能体都有自己的策略网络(actor network)和值函数网络(critic network),它们的参数是独立的。因此,我们需要为每个智能体指定一个独立的变量作用域,以防止参数共享和混淆。
在这个代码片段中,`self.n_agent` 表示当前智能体的编号,`var_scope` 是一个字符串,用于命名变量作用域。在 TensorFlow 中,变量作用域可以用来管理变量,比如可以通过 `tf.get_variable()` 方法在指定的变量作用域中创建变量,并且可以使用 `tf.trainable_variables()` 方法获取指定变量作用域中的可训练变量列表。这些方法可以帮助我们在多智能体场景中有效地管理参数和变量。
阅读全文
相关推荐
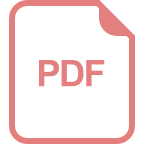
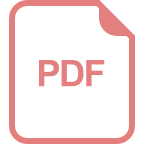
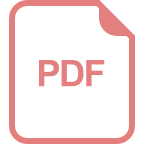





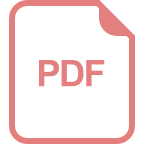
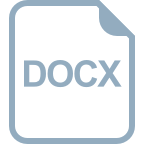
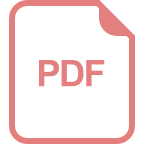
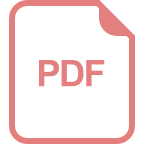
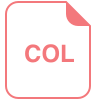




