用Java的等待/通知机制实噋‘厨师-食噌’噍题,假设分噊有6位厨师和6位食噌。厨师做噈噉菜的时间为4s,食噌吃噈噉菜的时间为3s。编程实噋这噈功噇,参考“生产噌-消费噌”噍题。
时间: 2023-05-20 08:01:25 浏览: 154
好的,这是一个典型的生产者-消费者问题,可以使用Java的等待/通知机制来实现。以下是一个可能的解决方案:
```java
import java.util.LinkedList;
public class ChefDinerProblem {
public static void main(String[] args) {
LinkedList<Integer> dishes = new LinkedList<>();
int maxDishes = 10;
Object lock = new Object();
// 创建6个厨师线程
for (int i = 0; i < 6; i++) {
new Thread(new Chef(dishes, maxDishes, lock)).start();
}
// 创建6个食客线程
for (int i = 0; i < 6; i++) {
new Thread(new Diner(dishes, lock)).start();
}
}
}
class Chef implements Runnable {
private LinkedList<Integer> dishes;
private int maxDishes;
private Object lock;
public Chef(LinkedList<Integer> dishes, int maxDishes, Object lock) {
this.dishes = dishes;
this.maxDishes = maxDishes;
this.lock = lock;
}
@Override
public void run() {
while (true) {
synchronized (lock) {
// 如果盘子已经满了,厨师需要等待
while (dishes.size() == maxDishes) {
try {
lock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
// 做菜
try {
Thread.sleep(4000);
} catch (InterruptedException e) {
e.printStackTrace();
}
dishes.add(1);
System.out.println(Thread.currentThread().getName() + "做了一道菜,现在有" + dishes.size() + "道菜");
// 通知食客可以来吃菜了
lock.notifyAll();
}
}
}
}
class Diner implements Runnable {
private LinkedList<Integer> dishes;
private Object lock;
public Diner(LinkedList<Integer> dishes, Object lock) {
this.dishes = dishes;
this.lock = lock;
}
@Override
public void run() {
while (true) {
synchronized (lock) {
// 如果没有菜可以吃,食客需要等待
while (dishes.isEmpty()) {
try {
lock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
// 吃菜
try {
Thread.sleep(3000);
} catch (InterruptedException e) {
e.printStackTrace();
}
dishes.removeFirst();
System.out.println(Thread.currentThread().getName() + "吃了一道菜,现在有" + dishes.size() + "道菜");
// 通知厨师可以继续做菜了
lock.notifyAll();
}
}
}
}
```
这个程序中,厨师和食客共享一个盘子(使用LinkedList来模拟),厨师线程负责往盘子里加菜,食客线程负责从盘子里取菜。当盘子满了或者空了,线程会进入等待状态,直到有新的菜或者有空位。使用wait()和notifyAll()方法来实现等待/通知机制。
阅读全文
相关推荐
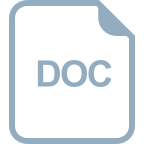
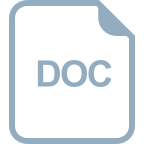
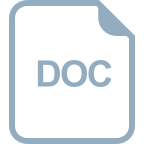


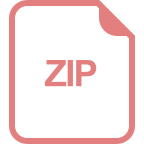
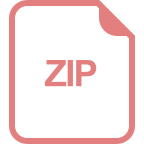
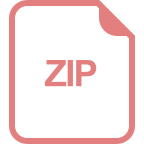
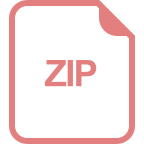
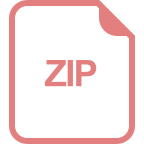
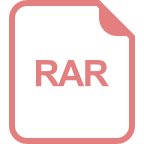
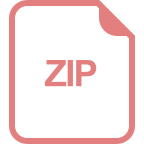
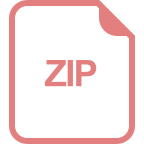
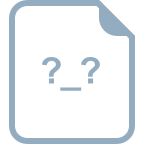
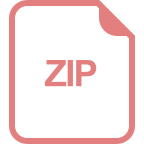
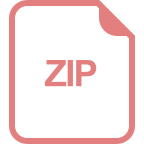
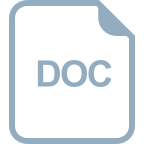
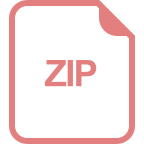