invalid indexer array, does not have integer dtype: array(0.)
时间: 2024-02-12 12:04:55 浏览: 54
This error message usually occurs when you are trying to use a non-integer value as an index to an array that requires integer indexing.
For example, if you have a NumPy array `arr` and you try to access an element using a non-integer value like `arr[0.]`, you will get this error.
To fix this error, you need to ensure that you are using integer values as indices. You could also check if the data type of your index array is integer using the `dtype` attribute.
Here is an example of how to reproduce this error:
```
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
index = 0.
arr[index] # This will raise a TypeError
```
In this example, the `index` variable is a float value of `0.`, which is not a valid integer index. To fix this error, you could cast the `index` value to an integer:
```
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
index = 0.
arr[int(index)] # Use integer indexing instead
```
Alternatively, you could define the `index` variable as an integer value in the first place:
```
import numpy as np
arr = np.array([1, 2, 3, 4, 5])
index = 0
arr[index] # Use integer indexing
```
I hope this helps!
相关推荐
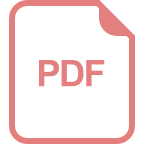
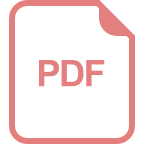














