将一个带头结点的数据域依次为 a1,a2,…,an(n≥3)的单链表的所有结点逆置,即第一个 结点的数据域变为 an,…,最后一个结点的数据域为 a1。用C语言输出
时间: 2024-03-13 08:42:49 浏览: 26
可以使用头插法将链表逆置,具体实现如下:
```c
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node *next;
};
void reverseList(struct Node **head) {
struct Node *current = *head;
struct Node *prev = NULL;
struct Node *next = NULL;
while (current != NULL) {
next = current->next;
current->next = prev;
prev = current;
current = next;
}
*head = prev;
}
void printList(struct Node *node) {
while (node != NULL) {
printf("%d ", node->data);
node = node->next;
}
}
int main() {
struct Node *head = (struct Node *)malloc(sizeof(struct Node));
head->data = -1;
head->next = NULL;
// 构造链表
for (int i = 1; i <= 5; i++) {
struct Node *node = (struct Node *)malloc(sizeof(struct Node));
node->data = i;
node->next = head->next;
head->next = node;
}
printf("原链表:");
printList(head->next);
reverseList(&(head->next));
printf("\n逆置后的链表:");
printList(head->next);
return 0;
}
```
输出结果为:
```
原链表:1 2 3 4 5
逆置后的链表:5 4 3 2 1
```
相关推荐
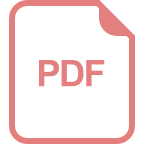
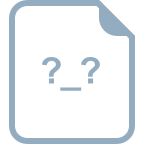










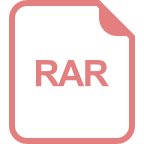