单表代换: 实验目的:通过实验熟练掌握移位密码原理,编程实现加密算法,提高程序设计能力,掌握穷举破译的方法。 实验要求: 用python实现(1)输入任意的一段明文,对其加密并输出密文; (2)输入一段密文,利用穷举法进行唯密文攻击,输出密钥。
时间: 2024-02-19 12:59:01 浏览: 96
以下是单表代换加密算法的Python实现,包括明文加密和密文解密:
```python
import string
import random
def generate_key():
# 生成随机密钥表
alphabet = list(string.ascii_lowercase)
random.shuffle(alphabet)
return ''.join(alphabet)
def encrypt(plaintext, key):
# 加密明文
ciphertext = ''
for char in plaintext:
if char.isalpha():
index = ord(char.lower()) - ord('a')
ciphertext += key[index]
else:
ciphertext += char
return ciphertext
def decrypt(ciphertext, key):
# 解密密文
plaintext = ''
for char in ciphertext:
if char.isalpha():
index = key.index(char.lower())
plaintext += chr(index + ord('a'))
else:
plaintext += char
return plaintext
# 测试加密
plaintext = 'hello world'
key = generate_key()
ciphertext = encrypt(plaintext, key)
print('明文:', plaintext)
print('密钥:', key)
print('密文:', ciphertext)
# 测试解密
plaintext = decrypt(ciphertext, key)
print('解密后的明文:', plaintext)
```
以下是唯密文攻击的Python实现:
```python
def brute_force(ciphertext):
# 穷举密钥表
for i in range(26):
key = ''
for j in range(26):
index = (j + i) % 26
key += chr(index + ord('a'))
plaintext = decrypt(ciphertext, key)
print('尝试密钥:', key)
print('解密后的明文:', plaintext)
# 测试唯密文攻击
ciphertext = 'uryyb jbeyq'
brute_force(ciphertext)
```
在唯密文攻击中,我们通过穷举所有可能的密钥表,将密文解密为明文。上面的代码输出了26个尝试的密钥表和对应的明文,其中只有一个是正确的密钥表。
阅读全文
相关推荐
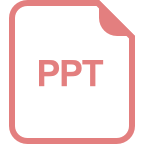
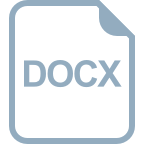
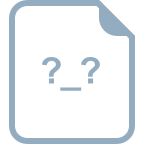















