windows下ZwQuerySystemInformation隐藏进程
时间: 2023-06-24 17:03:59 浏览: 190
在 Windows 系统下,可以使用 ZwQuerySystemInformation 函数来获取系统进程信息。但是,该函数只能获取到当前已经存在的进程信息,无法获取到已经隐藏的进程信息。因此,如果要获取隐藏进程信息,需要使用其他技术手段,比如 Hook 一些系统函数,或者使用 Rootkit 技术。但是,这些技术都是非常复杂和危险的,需要非常谨慎使用。同时,这些技术都可能被杀毒软件和系统安全机制检测到,从而导致被拦截和阻止。因此,在实际应用中需要慎重考虑使用这些技术。
相关问题
windows下ZwQuerySystemInformation隐藏进程,给出c++代码
以下是一个简单的 C++ 代码示例,演示了如何使用 `ZwQuerySystemInformation` 函数来遍历系统中的进程并隐藏指定进程。
注意:这仅是一个示例代码,仅供学习和参考。在实际使用中,请确保您已经理解代码的含义,并且不要滥用这个功能。
```cpp
#include <Windows.h>
#include <iostream>
typedef NTSTATUS(WINAPI* pfnZwQuerySystemInformation)(ULONG, PVOID, ULONG, PULONG);
int main()
{
// 要隐藏的进程名
const wchar_t* processName = L"Demo.exe";
// 加载 ntdll.dll 并获取 ZwQuerySystemInformation 函数地址
HMODULE ntdll = LoadLibrary(L"ntdll.dll");
pfnZwQuerySystemInformation ZwQuerySystemInformation = (pfnZwQuerySystemInformation)GetProcAddress(ntdll, "ZwQuerySystemInformation");
if (ZwQuerySystemInformation == nullptr)
{
std::cerr << "Failed to get ZwQuerySystemInformation address." << std::endl;
return 1;
}
// 获取系统进程信息
ULONG bufferSize = 0;
ZwQuerySystemInformation(SystemProcessInformation, nullptr, 0, &bufferSize);
PVOID buffer = VirtualAlloc(nullptr, bufferSize, MEM_COMMIT, PAGE_READWRITE);
if (buffer == nullptr)
{
std::cerr << "Failed to allocate memory." << std::endl;
return 1;
}
ULONG returnLength;
NTSTATUS status = ZwQuerySystemInformation(SystemProcessInformation, buffer, bufferSize, &returnLength);
if (status != STATUS_SUCCESS)
{
std::cerr << "Failed to get system process information." << std::endl;
VirtualFree(buffer, 0, MEM_RELEASE);
return 1;
}
// 遍历进程信息并隐藏指定进程
PSYSTEM_PROCESS_INFORMATION current = (PSYSTEM_PROCESS_INFORMATION)buffer;
PSYSTEM_PROCESS_INFORMATION previous = nullptr;
while (current != nullptr)
{
if (wcscmp(current->ImageName.Buffer, processName) == 0)
{
// 隐藏进程
if (previous != nullptr)
{
previous->NextEntryOffset += current->NextEntryOffset;
}
else
{
// 如果是第一个进程,则需要更新 ProcessInfoListHead 指针
((PSYSTEM_PROCESS_INFORMATION)buffer)->NextEntryOffset = current->NextEntryOffset;
}
}
// 移动到下一个进程
previous = current;
current = (PSYSTEM_PROCESS_INFORMATION)((PUCHAR)current + current->NextEntryOffset);
}
// 释放内存并退出
VirtualFree(buffer, 0, MEM_RELEASE);
return 0;
}
```
windows隐藏进程防检测
在 Windows 中,隐藏进程的方法有很多种,以下是其中一些常见的方法:
1. 修改进程名称:修改进程名称可以使进程不易被发现,可以使用一些工具如 PE Explorer 等来修改进程名称。
2. Hook 系统函数:通过 Hook 一些系统函数,可以使进程的存在得到隐藏,如 Hook ZwQuerySystemInformation 等函数。
3. 隐藏进程所在的驱动:有些进程是需要依赖驱动的,可以通过隐藏驱动的方式来隐藏进程,可以使用一些工具如 RootkitUnhooker 等来实现。
4. 注册表设置:通过修改注册表中的一些键值,可以使进程不被发现,如将 HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft\Windows NT\CurrentVersion\Image File Execution Options\ 这个键下的进程名称改为 Debugger 可以防止这个进程被启动。
5. 使用 Rootkit 技术:Rootkit 是一种可以隐藏进程的技术,它可以通过修改系统核心代码来实现进程的隐藏,但是这种技术比较危险,容易导致系统崩溃。
阅读全文
相关推荐
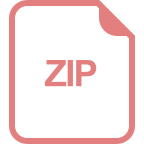
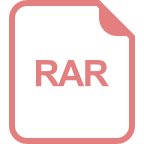
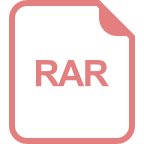
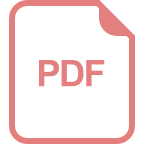
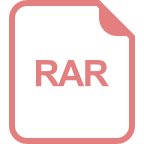
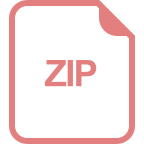
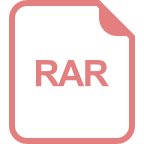
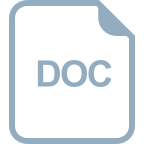
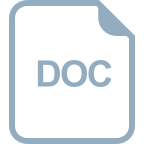
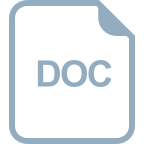
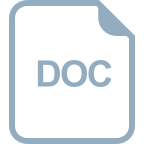
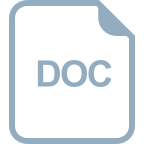
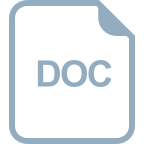
