unity3D项目当中,如何实现摄像机画面截图这个功能
时间: 2024-01-17 12:05:46 浏览: 35
在Unity3D中,可以使用RenderTexture和Texture2D来实现摄像机画面截图的功能。下面是一个基本的示例代码:
```csharp
public class CameraScreenshot : MonoBehaviour
{
public Camera screenshotCamera; // 需要截图的摄像机
public void TakeScreenshot()
{
// 创建RenderTexture
RenderTexture rt = new RenderTexture(Screen.width, Screen.height, 24);
// 设置摄像机的targetTexture为RenderTexture
screenshotCamera.targetTexture = rt;
// 渲染一帧画面
screenshotCamera.Render();
// 恢复摄像机的targetTexture
screenshotCamera.targetTexture = null;
// 将RenderTexture转换为Texture2D
Texture2D screenshot = new Texture2D(Screen.width, Screen.height, TextureFormat.RGB24, false);
RenderTexture.active = rt;
screenshot.ReadPixels(new Rect(0, 0, Screen.width, Screen.height), 0, 0);
screenshot.Apply();
RenderTexture.active = null;
// 保存截图
byte[] bytes = screenshot.EncodeToPNG();
File.WriteAllBytes(Application.dataPath + "/screenshot.png", bytes);
}
}
```
在这个示例代码中,我们创建了一个CameraScreenshot类,其中包括一个需要截图的摄像机和一个TakeScreenshot()方法。在TakeScreenshot()方法中,我们创建了一个RenderTexture,并将摄像机的targetTexture设置为这个RenderTexture,然后渲染一帧画面。接着,我们将RenderTexture转换为Texture2D,并保存为PNG格式的图片。注意,在保存图片时,需要使用File.WriteAllBytes()方法将图片数据写入磁盘。
需要注意的是,这个示例代码只是一个基本的实现方式,实际应用中可能需要根据具体需求进行修改。比如,可以在截图时添加水印、调整截图的分辨率等。此外,由于RenderTexture和Texture2D占用内存较高,建议在使用完毕后及时释放内存,以避免出现内存泄漏等问题。
相关推荐
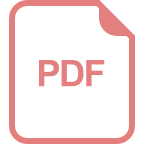
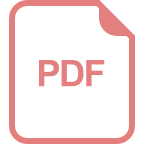
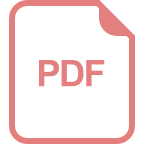














