c sharp窗口最大化用代码怎么实现
时间: 2024-06-10 17:06:23 浏览: 79
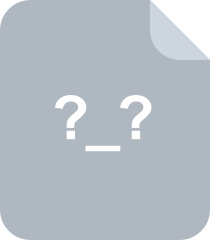
c#代码设计窗体最大化
您可以使用以下代码来实现C#窗口的最大化:
```
this.WindowState = FormWindowState.Maximized;
```
或者
```
this.FormBorderStyle = FormBorderStyle.None;
this.WindowState = FormWindowState.Maximized;
```
第一个代码片段将窗体状态设置为最大化,第二个代码片段还将边框样式设置为无边框,实现更完整的最大化效果。
阅读全文
相关推荐
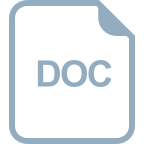
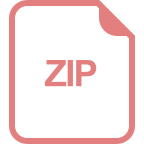
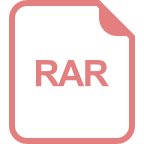
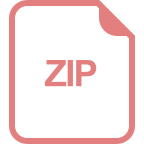
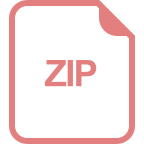
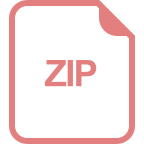
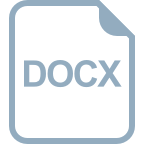
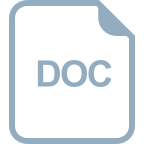
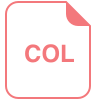
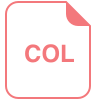
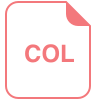
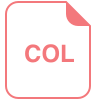
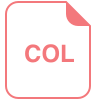
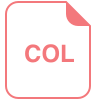