使用c++4.编写一个函数要求实现一个字符串压缩的过程。压缩规则是:如果某个字符x连续出现n(n>1)次,则将这n个字符x替换为nx的形式;否则保持不变。 函数声明可以为: void strzip(char* p,char *zp); 可以按如下形式设计程序: #include <iostream> using namespace std; void strzip(char* p,char *zp) { … } int main() { char str1[100] = "HelloWorldddddC++MATLABBBBBBBBBBB"; char str2[100]; strzip(str1, str2); cout <<str2<< endl; return 0; }
时间: 2024-01-29 09:02:32 浏览: 31
好的,我明白您的问题了。下面是实现字符串压缩的C++代码,希望能够帮助到您:
```c++
#include <iostream>
#include <cstring>
using namespace std;
void strzip(char* p, char* zp) {
int len = strlen(p);
int index = 0;
for (int i = 0; i < len; i++) {
int count = 1;
while (i < len - 1 && p[i] == p[i + 1]) {
count++;
i++;
}
if (count > 1) {
zp[index++] = p[i];
zp[index++] = '0' + count;
}
else {
zp[index++] = p[i];
}
}
zp[index] = '\0';
}
int main() {
char str1[100] = "HelloWorldddddC++MATLABBBBBBBBBBB";
char str2[100];
strzip(str1, str2);
cout << str2 << endl;
return 0;
}
```
解释如下:
1. `void strzip(char* p, char* zp)`:定义一个函数,接受两个指向字符数组的指针参数,分别是原始字符串和压缩后的字符串。
2. `int len = strlen(p)`:获取原始字符串的长度。
3. `for (int i = 0; i < len; i++)`:遍历原始字符串中的每一个字符。
4. `int count = 1`:初始化计数器为1。
5. `while (i < len - 1 && p[i] == p[i + 1])`:如果当前字符和下一个字符相同,则计数器加1,继续遍历下一个字符,直到不相同或已经遍历到字符串末尾。
6. `if (count > 1)`:如果计数器大于1,说明有连续的相同字符。
7. `zp[index++] = p[i]`:将当前字符存入压缩后的字符串中。
8. `zp[index++] = '0' + count`:将计数器转换为字符类型并存入压缩后的字符串中。
9. `else`:如果计数器等于1,说明当前字符不连续,直接存入压缩后的字符串中。
10. `zp[index] = '\0'`:在压缩后的字符串末尾添加一个空字符,表示字符串结束。
11. `char str1[100] = "HelloWorldddddC++MATLABBBBBBBBBBB"`:定义一个原始字符串。
12. `char str2[100]`:定义一个压缩后的字符串。
13. `strzip(str1, str2)`:调用`strzip`函数进行字符串压缩。
14. `cout << str2 << endl`:输出压缩后的字符串。
15. `return 0`:程序结束。
相关推荐
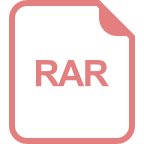
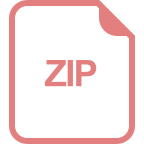
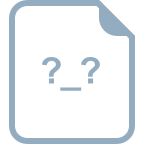














