c# 删除word空白页的所有示例
时间: 2023-06-23 08:49:43 浏览: 207
以下是一些示例代码,演示了如何在 C# 中删除 Word 文档中的空白页。
1. 使用 Microsoft.Office.Interop.Word 库
``` csharp
using Microsoft.Office.Interop.Word;
public void DeleteBlankPages(string filePath)
{
Application wordApp = new Application();
Document wordDoc = wordApp.Documents.Open(filePath);
int pageCount = wordDoc.ComputeStatistics(WdStatistic.wdStatisticPages, false);
int deletedPages = 0;
for (int i = pageCount; i >= 1; i--)
{
Range range = wordDoc.GoTo(WdGoToItem.wdGoToPage, WdGoToDirection.wdGoToAbsolute, i);
if (range.ComputeStatistics(WdStatistic.wdStatisticLines, false) == 0)
{
range.Delete();
deletedPages++;
}
}
wordDoc.Save();
wordDoc.Close();
wordApp.Quit();
Console.WriteLine($"Deleted {deletedPages} blank pages.");
}
```
2. 使用正则表达式
``` csharp
public void DeleteBlankPages(string filePath)
{
string pattern = "^\\s*$\n^\\s*$";
string replacement = "";
string text = File.ReadAllText(filePath);
text = Regex.Replace(text, pattern, replacement, RegexOptions.Multiline);
File.WriteAllText(filePath, text);
}
```
3. 使用 Aspose.Words 库
``` csharp
using Aspose.Words;
public void DeleteBlankPages(string filePath)
{
Document doc = new Document(filePath);
for (int i = doc.PageCount - 1; i >= 0; i--)
{
Section section = doc.Sections[i];
Body body = section.Body;
if (body.GetChildNodes(NodeType.Paragraph, true).Count == 0)
{
section.Remove();
}
}
doc.Save(filePath);
}
```
无论您选择哪种方法,都应该在删除空白页之前备份原始文档,以防止意外的数据丢失。同时,请注意,这些方法都可能会删除一些您并不想删除的内容,因此请谨慎使用。
相关推荐
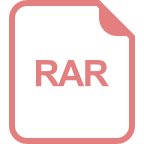
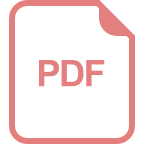














