使用python写一个线性回归实现波士顿房价预测
时间: 2023-10-16 09:31:36 浏览: 159
好的,下面是用Python实现波士顿房价预测的线性回归代码。
首先,我们需要加载数据集并进行预处理:
```python
import numpy as np
from sklearn.datasets import load_boston
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
# 加载数据集
boston = load_boston()
# 数据预处理
X = boston.data
y = boston.target
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
scaler = StandardScaler()
X_train = scaler.fit_transform(X_train)
X_test = scaler.transform(X_test)
```
接下来,我们可以实现线性回归模型:
```python
class LinearRegression:
def __init__(self, lr=0.01, n_iters=1000):
self.lr = lr
self.n_iters = n_iters
self.weights = None
self.bias = None
def fit(self, X, y):
n_samples, n_features = X.shape
# 初始化权重和偏差
self.weights = np.zeros(n_features)
self.bias = 0
# 梯度下降
for _ in range(self.n_iters):
y_pred = np.dot(X, self.weights) + self.bias
dw = (1 / n_samples) * np.dot(X.T, (y_pred - y))
db = (1 / n_samples) * np.sum(y_pred - y)
self.weights -= self.lr * dw
self.bias -= self.lr * db
def predict(self, X):
return np.dot(X, self.weights) + self.bias
```
最后,我们可以使用训练集拟合模型并在测试集上进行预测和评估:
```python
# 训练模型
model = LinearRegression(lr=0.01, n_iters=1000)
model.fit(X_train, y_train)
# 在测试集上进行预测和评估
y_pred = model.predict(X_test)
mse = np.mean((y_pred - y_test) ** 2)
rmse = np.sqrt(mse)
r2_score = 1 - (np.sum((y_test - y_pred) ** 2) / np.sum((y_test - np.mean(y_test)) ** 2))
print("MSE:", mse)
print("RMSE:", rmse)
print("R2 Score:", r2_score)
```
希望能对你有所帮助!
阅读全文
相关推荐
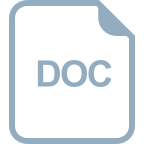
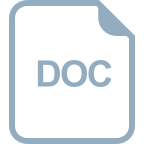
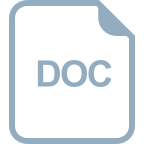
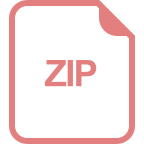
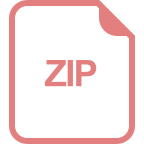
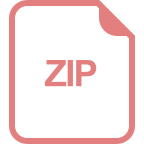
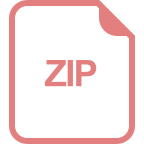



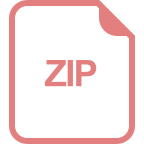
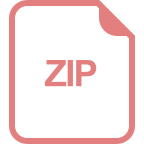
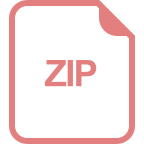
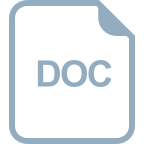




