java 设定时间 date格式
时间: 2023-08-05 09:44:00 浏览: 48
在Java中,可以使用Date类来表示时间。以下是使用Date类设定时间的示例代码:
```
import java.util.Date;
public class SetTimeExample {
public static void main(String[] args) {
// 获取当前时间
Date now = new Date();
System.out.println("当前时间为: " + now);
// 设定时间为 2021年12月31日 23:59:59
Date newTime = new Date(121, 11, 31, 23, 59, 59);
System.out.println("设定时间为: " + newTime);
}
}
```
在这个示例代码中,我们使用了Date类来表示时间。使用Date类设定时间的方法是通过构造函数来实现的。我们设定了一个新的时间,即2021年12月31日23时59分59秒。
需要注意的是,Date类的构造函数中,年份需要减去1900,月份需要减去1。这是由于Date类的设计历史问题造成的。另外,Date类由于存在线程安全问题,已经被Java8中的新日期时间API所替代,建议使用新API。
相关问题
java设置date的流逝时间
要设置Java中Date对象的流逝时间,你可以使用Java中的Calendar类来实现。下面是一个示例代码,它将创建一个Date对象并将其时间设置为当前时间,然后将流逝的时间设置为5分钟后的时间:
```java
import java.util.Calendar;
import java.util.Date;
public class Main {
public static void main(String[] args) {
Date now = new Date();
Calendar calendar = Calendar.getInstance();
calendar.setTime(now);
calendar.add(Calendar.MINUTE, 5);
Date future = calendar.getTime();
System.out.println(future);
}
}
```
在上面的代码中,我们首先创建一个Date对象来表示当前时间。然后,我们使用Calendar类将Date对象转换为日历对象,并使用setTime方法将其设置为当前时间。接下来,我们使用add方法将5分钟添加到日历对象中,并使用getTime方法将其转换回Date对象。最后,我们打印出结果,也就是5分钟后的时间。
java给date 设置指定时间
可以使用java.util.Date类的setTime()方法来设置Date对象的时间。该方法接受一个表示从1970年1月1日0时0分0秒开始经过的毫秒数的long类型参数。例如,以下代码将Date对象的时间设置为2021年7月1日9时30分0秒:
```
Date date = new Date();
date.setTime(1625110200000L);
```
其中,1625110200000L表示从1970年1月1日0时0分0秒开始到2021年7月1日9时30分0秒经过的毫秒数。你可以根据需要修改这个数值来设置任意时间。
相关推荐
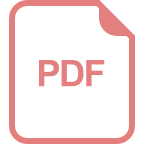












