利用自定义插件和组件实现一个简单的商品列表页,包含商品图、商品名、价格
时间: 2024-05-24 10:11:44 浏览: 194
和库存数量等信息。
首先,我们需要先定义一个商品列表组件,例如:
```html
<template>
<div class="product-list">
<div v-for="(product, index) in products" :key="index" class="product-item">
<img :src="product.image" alt="product image">
<h3 class="product-name">{{ product.name }}</h3>
<p class="product-price">{{ product.price }}</p>
<p class="product-stock">库存:{{ product.stock }}</p>
</div>
</div>
</template>
<script>
export default {
name: 'ProductList',
props: {
products: {
type: Array,
required: true
}
}
}
</script>
<style scoped>
.product-list {
display: flex;
flex-wrap: wrap;
justify-content: center;
align-items: center;
}
.product-item {
display: flex;
flex-direction: column;
align-items: center;
margin: 1rem;
padding: 1rem;
border: 1px solid #ccc;
border-radius: 0.5rem;
box-shadow: 0 0.25rem 0.5rem rgba(0, 0, 0, 0.1);
}
.product-name {
margin-top: 1rem;
font-size: 1.2rem;
font-weight: bold;
}
.product-price {
margin-top: 0.5rem;
font-size: 1.2rem;
}
.product-stock {
margin-top: 0.5rem;
font-size: 1rem;
color: #666;
}
</style>
```
这个组件接受一个名为 `products` 的数组类型的 prop,包含每个商品的信息,例如:
```js
[
{
id: 1,
name: '商品1',
image: 'https://example.com/product1.jpg',
price: 100,
stock: 10
},
{
id: 2,
name: '商品2',
image: 'https://example.com/product2.jpg',
price: 200,
stock: 20
},
// ...
]
```
接下来,我们可以定义一个插件来注册这个组件:
```js
import ProductList from './ProductList.vue'
export default {
install(Vue) {
Vue.component('product-list', ProductList)
}
}
```
然后在主入口文件中使用这个插件:
```js
import Vue from 'vue'
import App from './App.vue'
import ProductListPlugin from './ProductListPlugin'
Vue.use(ProductListPlugin)
new Vue({
render: h => h(App)
}).$mount('#app')
```
最后,在页面中使用这个组件即可:
```html
<template>
<div id="app">
<product-list :products="products"></product-list>
</div>
</template>
<script>
export default {
name: 'App',
data() {
return {
products: [
{
id: 1,
name: '商品1',
image: 'https://example.com/product1.jpg',
price: 100,
stock: 10
},
{
id: 2,
name: '商品2',
image: 'https://example.com/product2.jpg',
price: 200,
stock: 20
},
// ...
]
}
}
}
</script>
```
阅读全文
相关推荐
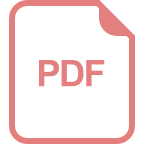


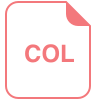
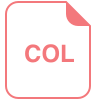
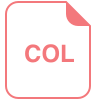
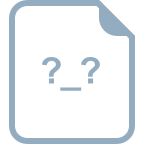
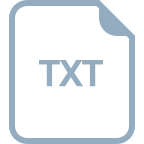
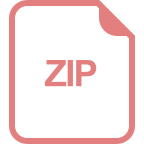
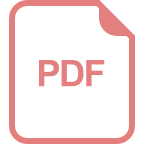
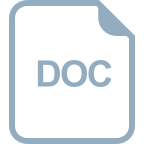
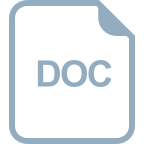
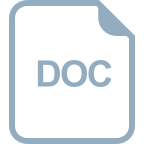
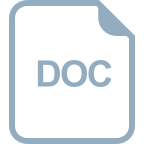
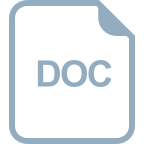
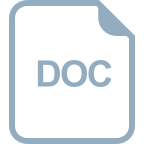
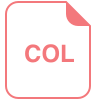