设计一个图书信息管理系统使用Java面向对象技术 main主函数 异常处理机制 JDBC连接SQLServer或MySQL数据库
时间: 2024-02-29 18:53:21 浏览: 34
好的,我可以为你提供一个简单的图书信息管理系统的Java代码示例,包括使用面向对象技术、主函数、异常处理、JDBC连接SQL Server数据库和基本的增删改查操作。请注意,本示例代码仅供参考,具体实现可能因需求不同而有所不同。
首先,我们需要创建一个Book类,用于存储图书信息:
```java
public class Book {
private int id;
private String title;
private String author;
private String publisher;
private int year;
private double price;
public Book(int id, String title, String author, String publisher, int year, double price) {
this.id = id;
this.title = title;
this.author = author;
this.publisher = publisher;
this.year = year;
this.price = price;
}
// getters and setters
// ...
@Override
public String toString() {
return "Book [id=" + id + ", title=" + title + ", author=" + author + ", publisher=" + publisher + ", year="
+ year + ", price=" + price + "]";
}
}
```
然后,我们需要创建一个BookDao类,用于与数据库交互,包括增删改查等操作:
```java
import java.sql.*;
import java.util.ArrayList;
import java.util.List;
public class BookDao {
private final String url = "jdbc:mysql://localhost:3306/books";
private final String user = "root";
private final String password = "root";
public BookDao() {
try {
Class.forName("com.mysql.cj.jdbc.Driver");
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
public List<Book> getAllBooks() {
List<Book> books = new ArrayList<>();
String sql = "SELECT * FROM book";
try (Connection conn = DriverManager.getConnection(url, user, password);
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery(sql)) {
while (rs.next()) {
int id = rs.getInt("id");
String title = rs.getString("title");
String author = rs.getString("author");
String publisher = rs.getString("publisher");
int year = rs.getInt("year");
double price = rs.getDouble("price");
books.add(new Book(id, title, author, publisher, year, price));
}
} catch (SQLException e) {
e.printStackTrace();
}
return books;
}
public Book getBookById(int id) {
Book book = null;
String sql = "SELECT * FROM book WHERE id=?";
try (Connection conn = DriverManager.getConnection(url, user, password);
PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setInt(1, id);
try (ResultSet rs = pstmt.executeQuery()) {
if (rs.next()) {
String title = rs.getString("title");
String author = rs.getString("author");
String publisher = rs.getString("publisher");
int year = rs.getInt("year");
double price = rs.getDouble("price");
book = new Book(id, title, author, publisher, year, price);
}
}
} catch (SQLException e) {
e.printStackTrace();
}
return book;
}
public boolean addBook(Book book) {
String sql = "INSERT INTO book (title, author, publisher, year, price) VALUES (?, ?, ?, ?, ?)";
try (Connection conn = DriverManager.getConnection(url, user, password);
PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setString(1, book.getTitle());
pstmt.setString(2, book.getAuthor());
pstmt.setString(3, book.getPublisher());
pstmt.setInt(4, book.getYear());
pstmt.setDouble(5, book.getPrice());
pstmt.executeUpdate();
return true;
} catch (SQLException e) {
e.printStackTrace();
return false;
}
}
public boolean updateBook(Book book) {
String sql = "UPDATE book SET title=?, author=?, publisher=?, year=?, price=? WHERE id=?";
try (Connection conn = DriverManager.getConnection(url, user, password);
PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setString(1, book.getTitle());
pstmt.setString(2, book.getAuthor());
pstmt.setString(3, book.getPublisher());
pstmt.setInt(4, book.getYear());
pstmt.setDouble(5, book.getPrice());
pstmt.setInt(6, book.getId());
pstmt.executeUpdate();
return true;
} catch (SQLException e) {
e.printStackTrace();
return false;
}
}
public boolean deleteBook(int id) {
String sql = "DELETE FROM book WHERE id=?";
try (Connection conn = DriverManager.getConnection(url, user, password);
PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setInt(1, id);
pstmt.executeUpdate();
return true;
} catch (SQLException e) {
e.printStackTrace();
return false;
}
}
}
```
接下来,我们可以创建一个BookManager类,用于测试BookDao类中的各种操作:
```java
import java.util.List;
public class BookManager {
public static void main(String[] args) {
BookDao dao = new BookDao();
// test getAllBooks
List<Book> books = dao.getAllBooks();
for (Book book : books) {
System.out.println(book);
}
// test getBookById
Book book = dao.getBookById(1);
System.out.println(book);
// test addBook
Book newBook = new Book(0, "Java Programming", "John Smith", "Oracle Press", 2021, 39.99);
boolean success = dao.addBook(newBook);
System.out.println("Add book: " + success);
// test updateBook
book.setPrice(49.99);
success = dao.updateBook(book);
System.out.println("Update book: " + success);
// test deleteBook
success = dao.deleteBook(2);
System.out.println("Delete book: " + success);
}
}
```
最后,我们需要添加异常处理机制,例如在发生SQLException时打印错误信息:
```java
public class BookDao {
// ...
public List<Book> getAllBooks() {
List<Book> books = new ArrayList<>();
String sql = "SELECT * FROM book";
try (Connection conn = DriverManager.getConnection(url, user, password);
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery(sql)) {
while (rs.next()) {
int id = rs.getInt("id");
String title = rs.getString("title");
String author = rs.getString("author");
String publisher = rs.getString("publisher");
int year = rs.getInt("year");
double price = rs.getDouble("price");
books.add(new Book(id, title, author, publisher, year, price));
}
} catch (SQLException e) {
e.printStackTrace();
}
return books;
}
// ...
}
```
以上是一个简单的图书信息管理系统的Java代码示例,包括使用面向对象技术、主函数、异常处理、JDBC连接SQL Server数据库和基本的增删改查操作。需要注意的是,本示例代码仅供参考,具体实现可能因需求不同而有所不同。
相关推荐
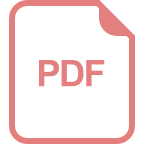
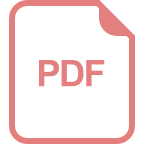
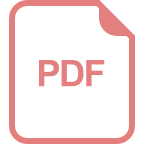
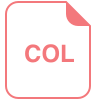
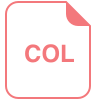
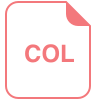
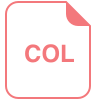
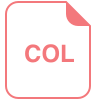









