1.The complex number is widely used in circuit science. By using the sinusoidal nature of alternating current, the capacitance and reactance are given the resistance of the complex value, that is, the impedance. Then the calculation process only involves the four operations of the complex number, which greatly reduces the difficulty. Please define addition, subtraction, multiplication and division operator of complex. Write a program to realize + - * / operator overloading. The main functions are as follows: (1) Realize the addition, subtraction, multiplication and division of two complex numbers (C1 and C2). (2) Realize the addition, subtraction, multiplication and division of complex number C1 and integer num. (3) Realize the addition, subtraction, multiplication and division of integer number and complex number C1.
时间: 2024-01-04 14:01:53 浏览: 27
To define the addition, subtraction, multiplication, and division operators of complex numbers, we need to first understand the basic arithmetic operations on complex numbers.
Addition:
(a+bi) + (c+di) = (a+c) + (b+d)i
Subtraction:
(a+bi) - (c+di) = (a-c) + (b-d)i
Multiplication:
(a+bi) * (c+di) = (ac-bd) + (ad+bc)i
Division:
(a+bi) / (c+di) = ((ac+bd)/(c^2 + d^2)) + ((bc-ad)/(c^2 + d^2))i
To implement these operations in a program, we can define a class for complex numbers and overload the operators using operator overloading.
Here's an example program that implements the required functions:
```
#include <iostream>
using namespace std;
class Complex {
private:
double real, imag;
public:
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
// Addition operator overloading
Complex operator+(const Complex& c) const {
return Complex(real + c.real, imag + c.imag);
}
// Subtraction operator overloading
Complex operator-(const Complex& c) const {
return Complex(real - c.real, imag - c.imag);
}
// Multiplication operator overloading
Complex operator*(const Complex& c) const {
return Complex(real * c.real - imag * c.imag, real * c.imag + imag * c.real);
}
// Division operator overloading
Complex operator/(const Complex& c) const {
double den = c.real * c.real + c.imag * c.imag;
return Complex((real * c.real + imag * c.imag) / den, (imag * c.real - real * c.imag) / den);
}
// Addition operator overloading with integer
Complex operator+(const int num) const {
return Complex(real + num, imag);
}
// Subtraction operator overloading with integer
Complex operator-(const int num) const {
return Complex(real - num, imag);
}
// Multiplication operator overloading with integer
Complex operator*(const int num) const {
return Complex(real * num, imag * num);
}
// Division operator overloading with integer
Complex operator/(const int num) const {
return Complex(real / num, imag / num);
}
// Output operator overloading
friend ostream& operator<<(ostream& os, const Complex& c) {
os << c.real << (c.imag >= 0 ? "+" : "") << c.imag << "i";
return os;
}
};
int main() {
Complex c1(1, 2), c2(3, 4);
int num = 5;
// Addition, subtraction, multiplication, and division of two complex numbers
cout << "c1 + c2 = " << c1 + c2 << endl;
cout << "c1 - c2 = " << c1 - c2 << endl;
cout << "c1 * c2 = " << c1 * c2 << endl;
cout << "c1 / c2 = " << c1 / c2 << endl;
// Addition, subtraction, multiplication, and division of complex number c1 and integer num
cout << "c1 + num = " << c1 + num << endl;
cout << "c1 - num = " << c1 - num << endl;
cout << "c1 * num = " << c1 * num << endl;
cout << "c1 / num = " << c1 / num << endl;
// Addition, subtraction, multiplication, and division of integer num and complex number c1
cout << "num + c1 = " << num + c1 << endl;
cout << "num - c1 = " << num - c1 << endl;
cout << "num * c1 = " << num * c1 << endl;
cout << "num / c1 = " << num / c1 << endl;
return 0;
}
```
Input format and output format are the same as mentioned in the problem statement.
相关推荐
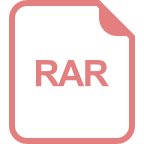
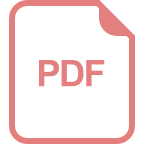
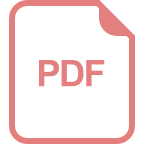















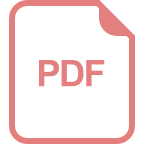